Introduction to Java
Ever wondered why Java's logo is a steaming cup of coffee? The creators of Java, while brainstorming a name for their new language, chose 'Java', a slang term for 'coffee'. Just as coffee fuels our day, Java powers the tech world with its robust and versatile features.
In this topic, we will explore why Java has been a popular choice among developers for over two decades and how it has brewed success in various domains. We will also introduce you to your very first Java program. So, grab your cup of coffee and join us on this exciting journey into the world of Java!

What is Java
Java is a high-level, class-based, object-oriented programming language. James Gosling at Sun Microsystems (now part of Oracle Corporation) designed it, and it was released in 1995. The language was developed with the "Write Once, Run Anywhere" (WORA) philosophy. This principle underscores Java's key feature - platform independence, allowing the same Java program to run on multiple platforms without modifications.
Java is designed to be both simple and powerful. It borrows its syntax from C and C++, but eliminates certain low-level programming complexities, such as explicit memory management and multiple inheritance found in C++. Unlike these two languages, Java does not require you to manually clean the application memory, as it has a garbage collector that performs this task automatically. Known for its robustness, security, and simplicity, Java has become a popular choice among developers worldwide. It supports different programming techniques, including generic programming, multithreaded and concurrent programming, and functional programming.
Where is Java Applied
Let's go through a typical day and see how Java impacts our lives without us even realizing it.
Imagine waking up to your Android alarm. As you reach out to snooze it, you're interacting with an application built using Java. You decide to work on a project using a development tool like IntelliJ IDEA or Eclipse. As you write and compile your code, Java is there, forming the backbone of these development tools. During lunch, you enjoy a Netflix show or Spotify music, both services powered by Java. Later, you finish the project and receive payment. Behind the scenes, Java is working diligently, processing your request. In the evening, you unwind with a game of Minecraft, yet another Java-based application.
Java is like a silent friend, aiding us and making our lives easier in numerous ways, from the moment we wake up till we call it a day.
A sample of Java
Let's create the classic "Hello, World!" program, a friendly greeting from your computer.
Here's the program:
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
Don't worry if it looks a bit cryptic now. You'll get the hang of it soon.
This program simply prints the phrase "Hello, World!" to the console. But there's a lot going on here:
- public class HelloWorld — In Java, all the code you write will be inside classes. We're telling Java we're creating a new public class (a kind of blueprint) and we're naming it HelloWorld. Every Java application has to have at least one class.
- public static void main(String[] args) — This is the heart of our program, where the execution begins.
- System.out.println("Hello, World!"); — These are our program's first words! This command instructs Java to print "Hello, World!" to the console via the
System.out.println()
method, providing instant feedback.
Note that in Java code, we use different types of brackets, and if you open any bracket, you are required to close it.
And there you have it, your first Java program! It's a modest step, but it marks the beginning of an exciting journey into Java programming.
Basic Literals
Any program, regardless of its complexity, always performs operations on numbers, strings, and other values. These values are called literals. There are many different sorts of literals in Java, but in this topic we will focus only on a few of them: the ones that surround us all the time in everyday life.
Basic literals in Java
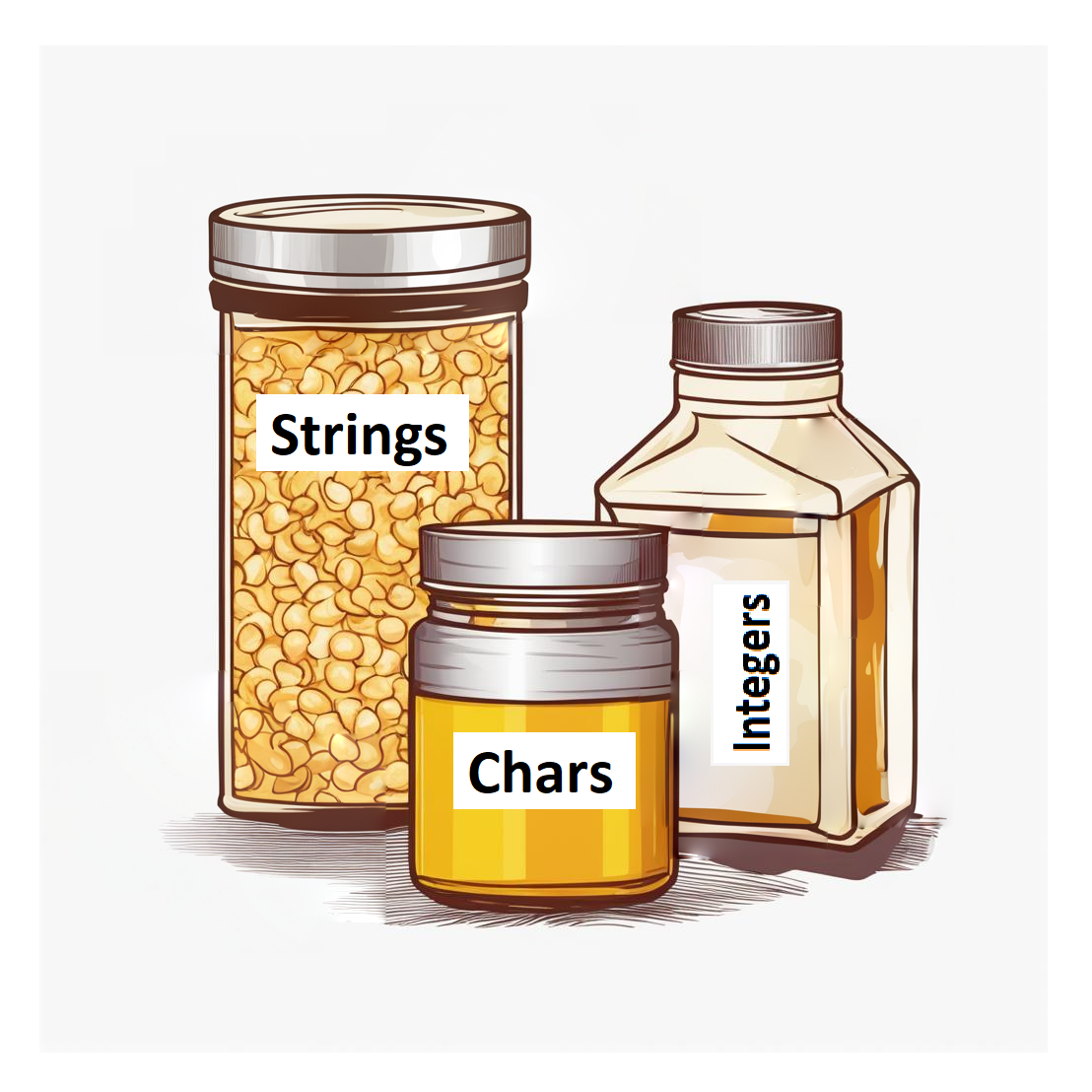
Consider literals as groceries. In order to use them, usually you need to store them somewhere. Typically, they are stored in variables, which you can think of as containers designed to hold a specific type of data.
However, variables can only store matching data. You wouldn't want to accidentally put honey in a cardboard cereal box or pour cereal into a salt shaker. To prevent such mistakes, learn to distinguish between the basic literals: integer numbers, strings, and characters.
Integer numbers
You use these numbers to count things in the real world just like you would use natural numbers. On top of this, integer numbers also include zero and negative numbers. Here's a list of valid integer number literals separated by commas: 0, 1, 2, 10, 11, -100
.
Here is how you can use integers in your Java code:
int numApples = 1000;
Reading code is crucial for anyone working in as a programmer, so let's untangle the example together. Here you put the integer 1000 into a variable of an integer type, called numApples
. This is similar to filling a container with its designated contents!
You can increase code readability by dividing the digit into blocks with underscores: 1_000_000
is more readable than 1000000
. And don't worry, the underscore symbol will not impact our original number.
So let's pack our apples to make selling them easier:
int numPackedApples = 1_000_000;
Fear not if these code snippets aren't 100% clear to you yet! They aim to help you develop the skill of code reading. Just grasp the overall meaning and follow your study plan, and you'll be writing your own code in no time!
Characters
A character is a single symbol, enclosed in single quotes. You can use character literals to represent single letters like 'A'
or 'x'
, digits from '0'
to '9'
, whitespaces (' ')
, and other characters or symbols like '$'
.
You might be wondering, if both integer literals and character literals can represent digits, then how can we differentiate between them? The two literals are differentiated on the basis of single quotes. Take a look at how these two literals are different in code.
char charOne = '1'int numOne = 1
Fun fact: Characters sit between integers and strings: they resemble strings, yet you can do math with them. Each character corresponds to a unique numeric value that is used to identify it. We'll dive into this in a later topic.
Strings
A string is a sequence of characters, enclosed by double quotes. It represents text-based information, such as an advertising line, a webpage address, or a website login name. Here are some valid examples: "text"
, "I want to know Java"
, "123456"
, "[email protected]"
. As you can see, a string can include letters, digits, whitespaces, and other characters altogether.
A string consisting of a single character like "A"
is also a valid string, but do not confuse it with the 'A'
character. Note the difference in quotes!
char singleQuotedCharacter = 'A';
String doubleQuotedString = "A";
Printing literals using variables
In Java, assigning literals to variables and printing them using System.out.println()
is a fundamental operation. To assign a literal to a variable, you first declare the variable with its type and then initialize it with a literal value. For instance, you might declare an integer variable like int number = 42;
, where 42
is the literal value assigned to number
. Similarly, for a string variable, you could write String greeting = "Hello";
, where "Hello"
is the string literal assigned to greeting
. Once the variables are initialized, you can use System.out.println()
to print their values to the consolе:
public class Main {
public static void main(String[] args) {
int number = 42;
String greeting = "Hello";
System.out.println(number);
System.out.println(greeting);
}
}
For example, System.out.println(number);
will output the value 42
, and System.out.println(greeting);
will display Hello
. This approach allows you to store and manipulate literal values in variables and then output them as needed for various purposes in your Java programs.
The Hello World program
Here is the Java code of this program:
public class Main {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
You can type this code into an online code editor and then press the execute button. In the result section, you will see:
Hello, World!
If you have already installed Java, you can run the program on your computer. If you don't have Java installed on your device, there is no need to install it right now. We will come back to it later.
The basic terminology
Now that you have grasped the fundamental concept of Java programming, let's learn some basic terminology and then try to understand this program.
- Program — a sequence of instructions (called statements), which are executed one after another in a predictable manner. Sequential flow is the most common and straightforward sequence of statements, in which statements are executed in the order that they are written – from top to bottom in a sequential manner;
- Statement — a single action (like printing some text or arithmetic operation) terminated by a semicolon (
;
); - Block — a section of code enclosed in a pair of braces
{...}
; There are two such blocks in the program above; - Method — a block of code that, when called, performs specific actions mentioned within it (also known as a subprogram or procedure);
- Syntax — a set of rules that define how a code needs to be written in order to be valid; Java has its own specific syntax that we will explore further.
- Keyword — a word that has a special meaning in the programming language (
public
,class
, and many others). These words cannot be used as variable names in your own program; - Identifier or name — a word that refers to something in a program (such as a variable or a function name);
- Comment — a textual explanation of what the code does. Java comments start with
//
or are enclosed within/*
and*/
. Comments are ignored during program execution; - Whitespace — all characters that are not visible (space, tab, newline, etc.).
The Hello World program under a microscope
The Hello World program illustrates the basic elements of Java programs. For now, we will discuss only the most important elements.
1. The public class — It is the basic unit of a program. Every Java program must have at least one class. The definition of a class consists of the class
keyword followed by the class name. A class can have any name, such as App
, Main
, or Program
, but it must not start with a digit. A set of braces {...}
encloses the body of a class.
public class Main {
// A class can contain methods and statements
}
The text after //
is just a comment, not a part of the program. We will learn about comments in detail in later topics.
2. The main method — It is the entry point for a Java program. To make the program runnable, we put a method named main
inside the Main
class, otherwise, it will not run. Again, the braces {...}
enclose the body of the method, which contains programming statements.
public static void main(String[] args) {
// Statements go here
}
The keywords public
, static
, and void
will be discussed later, so just remember them as a basic syntax for now. The name of this method (main
) is predefined and should always be the same. Capitalization matters: if you name your first method Main, MAIN or something else, the program cannot start.
The element String[] args
represents a sequence of arguments passed to the program from the outside world. Don't worry about them right now as we will explore them in detail later.
3. Printing "Hello, World!" — The body of the method consists of programming statements that determine what the program should do after starting. Our program prints the string "Hello, World!" using the following statement:
System.out.println("Hello, World!"); // each statement has to end with ;
This is one of the most important things to understand from the Hello World program. We invoke a special method println()
to display a string followed by a new line on the screen. We will often use this approach to print something of interest to the screen. The text is printed without double quotes as ""
is only used to denote a string.
It is important to note that "Hello, World!" is not a keyword or an identifier; it is just some text to be printed.
Keywords
As you can see, even a simple Java program consists of many elements, including keywords that are parts of the language. In total, Java provides more than 50 keywords which you will gradually learn on this platform. The full list is here, though you don't need to remember all of them at this moment.
Note, main
is not included in the given list because it is not a keyword.
Conclusion
Java, known for its robust features and platform independence, ranks as one of the most popular and in-demand programming language. Its clear syntax and automatic memory management contribute to its popularity. As you progress in your learning, you'll explore Java's key concepts and methods more deeply. With Hyperskill to guide you, you're on your way to doing great things with Java!