SQL and Python: applying programming languages in machine learning, software development, and science careers
SQL and Python are two of the most popular programming languages. They have been consistently ranked among the top 10 essential tools by Stack Overflow, TIOBE, and IEEE Spectrum.
SQL, which stands for Structured Query Language, is the standard language with a simple syntax for database administrators to work with querying open-source relational database management systems: from statistical analysis to iterative data exploration. Python is a general-purpose programming language used in various tasks: from web application development process to machine learning, from mobile applications to refining artificial intelligence.
SQL and Python can handle complex queries and aggregate functions, but Python has a broader range of applications than SQL. In this article, we will examine the similarities and differences between the two languages and showcase how they can complement each other in different operations.
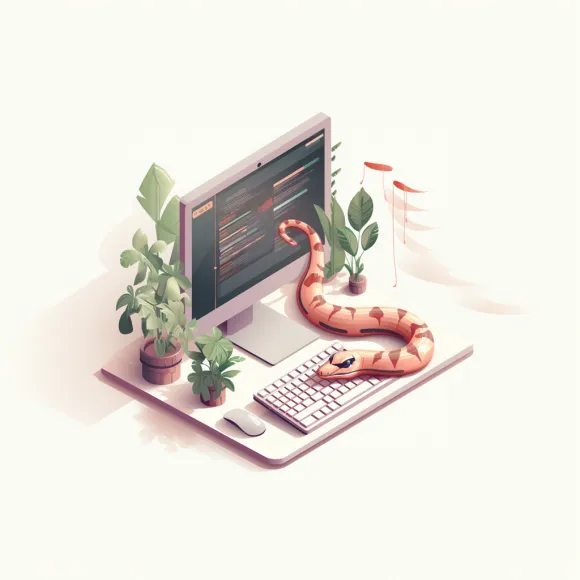
Similarities and Differences in Code
SQL and Python are some of the most popular programming languages for data-related tasks. SQL is listed in most data analyst job postings. Basic knowledge of SQL and visualization tools like Tableau and Power BI can get you started in data analytics. SQL and Python are suitable for data analysis, higher-level data manipulation, and visualization. You may need to integrate SQL with visualization tools, but Python has its visualization libraries.
SQL is significant when performing basic to medium computations to get quick data insights. Python is ideal when you want to transform your data and perform more advanced computations like regression tests and classification. Python is also better for business Intelligence and data mining, where you discover patterns and knowledge from large and complex datasets.
A key difference between SQL and Python is their different programming paradigms. SQL is a declarative programming language focusing on the database programming paradigm, while Python is a high-level language of the Object-oriented programming paradigm. In SQL, you specify what you want to happen, and the relational database management systems (DBMS) figure out how to do it for you. You must specify exactly how you want it to happen in Python step-by-step.
SQL and Python differ in the types of data structures they support. SQL primarily works with relational databases, tables organized into rows and columns, making it best suited for structured datasets.
In contrast, Python is more versatile and can handle a broader range of data structures, including dictionaries, queues, heaps, and trees.
Several implementations or “flavors” of the Python and SQL languages exist. There are the CPython, JPython, and IronPython implementations for Python, and there are the SQLite, MySQL, and PostgreSQL implementations for SQL.
Despite their differences, SQL and Python can be complementary. For example, SQL can extract data from relational databases for analysis and visualization in Python, and Python can preprocess data before storing it in a relational database.
Running SQL Queries from Scratch in Python
In this section, we will see how SQL and Python can complement each other for data-related and analysis tasks. Imagine the following Entity-Relationship Diagram (ERD):
You can create the relationship between the database table and the CREATE TABLE command in SQL. The data for the single database has been carefully organized in the Python dictionary, database_dict:
Next, we will use the Sqlite3 API to connect to the database. If the database does not exist, it will be. Otherwise, we'll establish a connection for a new session. The next step is to create a cursor object that can execute SQL commands in the session:
After executing the SQL commands and making changes to the database, the changes are not automatically saved to the database file stored on your drive. To make your changes permanent, commit your changes and close the connection to end the session.
You can also connect your database file with the sqlalchemy. Let’s execute the queries to confirm that our database has been populated with the data with this API:
You have successfully retrieved information from the students table in the school.db. Feel free to use the sqlite3 or sqlalchemy API to execute your queries.
Database Data Manipulation
SQL can also modify the data in huge databases. Let’s assume you made an incorrect entry for Jane Doe in the students table. The correct entry is Kate Doe, so let us update the necessary tables with this information:
You can verify that changes have been made by querying the students and student_info tables:
Great news! Your changes have been implemented successfully. Here's another example: if you need to obtain information about students' performance, you can use an SQL query to generate a Python DataFrame that displays their grades.
You can store the Python DataFrame as the students_gpa table in the school database as follows:
Data Analysis with SQL and Python
Data analysis software can be developed using both SQL and Python. SQL is commonly used for simple applications and queries such as calculating sums, averages, and counts. However, Python, with its dynamic typing, is better suited for more advanced scientific computing.
Let’s first see the distribution of grades by joining the students and students_gpa tables:
Next let’s see the students’ total scores across three subjects:
Next let’s see the course average:
In the next section, you will see how to visualize the query outputs with the Python Matplotlib’s library.
Visualization with Python Matplotlib
Data visualization is the process of presenting the results of your analyses in a graphical or visual format to help others better understand and interpret your results. Python has over 130,000 library options, especially for data visualization, but you may need to connect SQL database engines with visualization tools.
In this section, let's visualize the results of your data analyses. Let’s start by visualizing a bar chart of the students’ total scores:
Next, let’s visualize the course average scores using a pie chart:
Conveniently, you have been able to visualize the results of your analyses in Python with the matplotlib library.
Conclusion
To excel in data-related technical tasks, learning SQL and Python programming languages is crucial. These tools complement each other despite some differences, as highlighted in this article. Whether you aim to become a software engineer, machine learning engineer, or product manager, learning these fundamental languages is essential.
At Hyperskill, we offer a SQL with Python track that teaches you how to use SQL and Python to create programming projects. Additionally, we have a project-free SQL for Backend Developers track that allows you to efficiently master database theory and gain hands-on experience with MySQL. If you're interested in learning a high-level programming language like Python, we have several Python tracks available for beginners, each equipped with different tools.
Related Hyperskill Topics
like this