Setting up Python Development Environment for Beginners
Python is the most popular programming language for beginners learning to code. It is a beginner-friendly programming language because it is simple to set up and run, and code is easy to read and understand because its syntax is designed to resemble the English language, making it intuitive for beginners. It is also versatile, powering technologies like web applications, artificial intelligence, and automation.
This tutorial helps beginners get started with Python. You’ll learn how to download and install Python, as well as the IDLE and PyCharm IDEs. You’ll explore Python’s basic syntax and data types, and learn how to read, understand, and debug error messages. You’ll discover why Python is one of the most-loved programming languages and begin exploring the limitless possibilities the language offers.

Setting Up Python
Setting up Python and your development environment is the first step in your programming journey. The versions of Python and dependencies will be different for your projects. It is important to start learning how to manage your development environment from the get go. Virtual environments allow you to create isolated development environments where you can install specific packages and Python versions without affecting the global Python environment. Let’s get started by downloading and installing the latest version of Python:
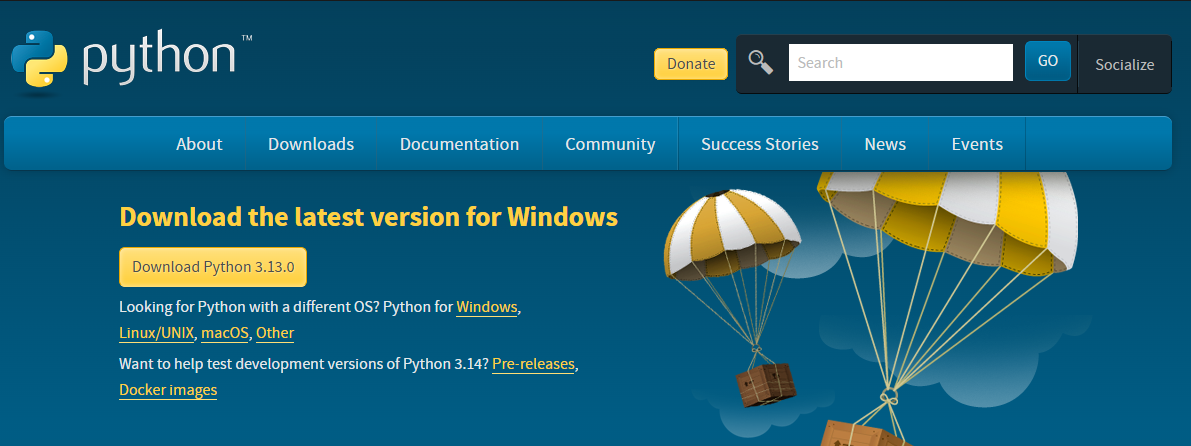
Next, create a directory or folder called Getting-Started and navigate in the command line to this folder. Before setting up your virtual environment, check the versions of Python that are already installed on your system:
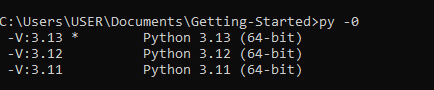
When setting your virtual environment, you can specify the version of Python you want to use. Not specifying the version will use the selected (represented with a *). Set up and activate the virtual environment named getStarted:

The (getStarted) indicates the virtual environment is activated. While a virtual environment is activated, pip will install packages into that specific environment. You can confirm the version of Python running in the virtual environment:

You can also run pip freeze to verify that no packages have been installed in the virtual environment. PyCharm simplifies the process of setting up and activating a virtual environment. When you create a new project, it automatically prepares the virtual environment for you:

PyCharm creates a virtual environment named .venv and activates it. You can confirm with pip freeze that no packages has been installed in this environment:
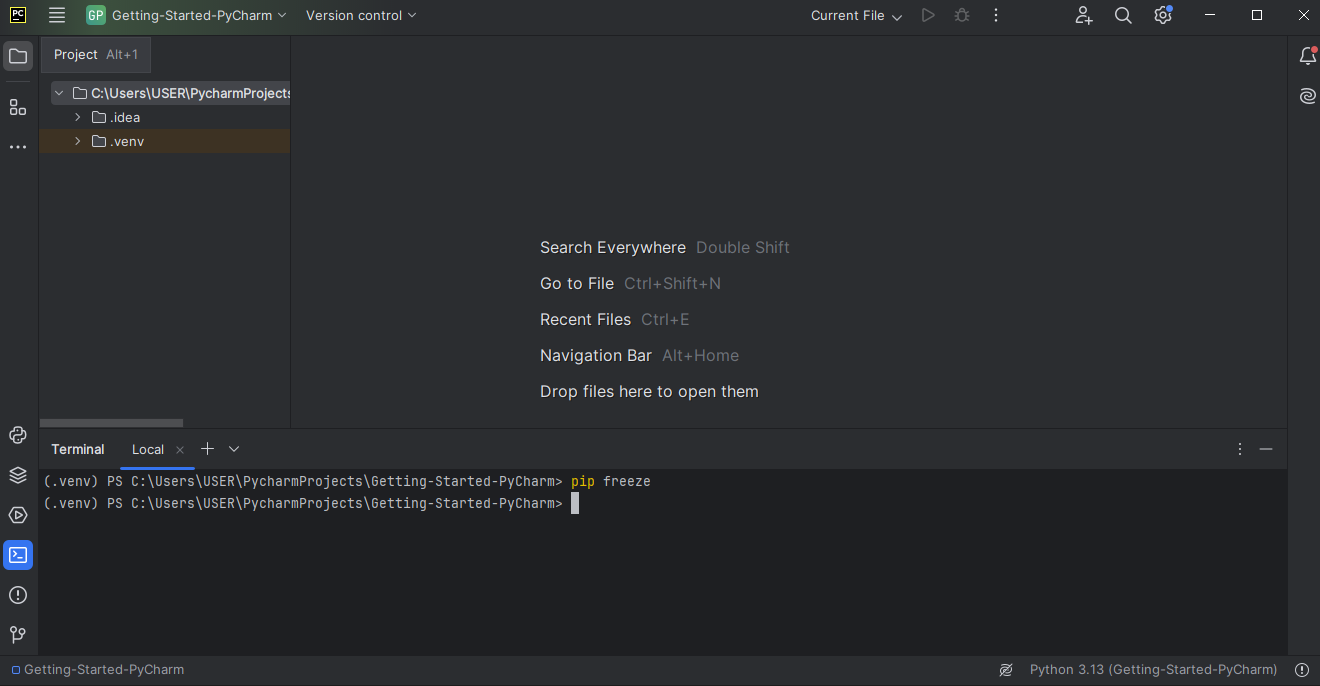
We will continue this tutorial with the PyCharm IDE because it allows us to easily set up our development environment.
Python program, variables, and operators
A Python program consists of a series of instructions stored in a .py file. When you run the program, Python processes these instructions line by line to execute the specified tasks. Programs often involve assigning values to variables, which act as named containers for storing data in memory. This allows you to reuse and manipulate the data throughout the program. In Python, you create variables by assigning values using the = operator.
# Assigning values to a variable
language = "Python"
print(language)
Variables in Python must start with a letter or underscore, followed by letters, numbers, or underscores. Python is dynamically typed, meaning there is no need to explicitly declare the variable type.
Python provides support for three primary numeric data types: integers, floating-point numbers, and complex numbers. The integer type, represented as int, is used for whole numbers without any fractional components, making it ideal for counting or indexing purposes. Floating-point numbers, denoted as float, handle numbers with decimal points, enabling precise representation of quantities like measurements or scientific calculations. For scenarios involving numbers with both real and imaginary parts, Python offers the complex type. This type is particularly useful in fields like engineering and physics, where complex numbers are often employed to model real-world phenomena:
# int
age = 25
# float
price = 7.99
# complex
z = 2 + 3j
Python also provides several data types for handling various kinds of information. The str type is used to represent text, making it essential for working with strings of characters such as words, sentences, or any textual data. The bool type, or boolean, is designed for representing truth values, specifically True and False, which are pivotal in logical operations and decision-making processes:
# str
greeting = "Hello"
# bool
is_completed = True
For organizing collections of items, Python offers the list and tuple types. A list is an ordered and mutable collection, allowing you to add, remove, or modify elements as needed. In contrast, a tuple is also an ordered collection but is immutable, meaning its elements cannot be changed once defined, which ensures consistency and reliability. Python also includes the dict type, or dictionary, which stores data as key-value pairs. This structure is especially useful for organizing and accessing data efficiently, as each key serves as a unique identifier for its associated value:
# list
languages = ["Python", "Kotlin", "Java"]
# tuples
coordinates = (3.5, 8.7)
# dictionary
user = {"name": "John Doe", "age": 25}
Let’s give this a try in PyCharm. Start by creating a new file named first_program.py. Enter the code provided below into the file, and once you’re finished, click the green play button to run it:
name = input("What is your name? ")
language = input("What is your favorite programming language? ")
print(f"\n{name}'s favorite programming language is {language}")
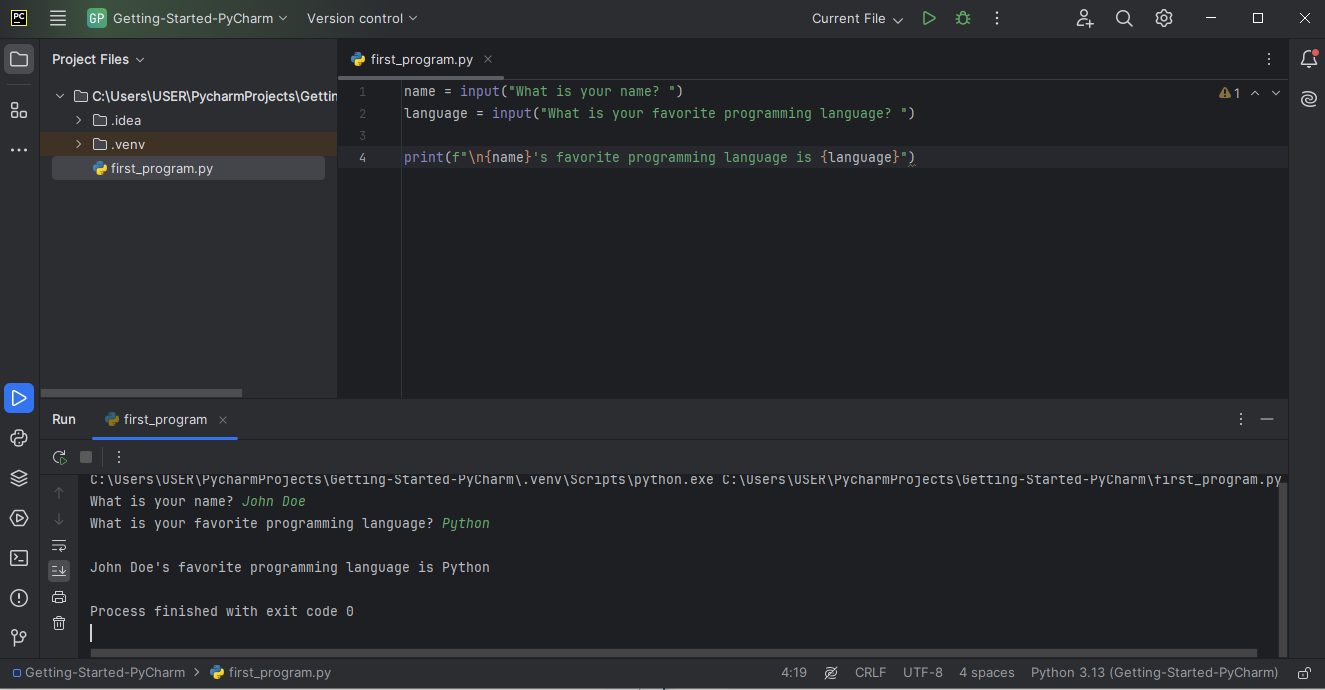
In Python, you can perform arithmetic operations with the arithmetic operators:
x = 10
y = 3
# Addition
print(x + y)
# subtraction
print(x - y)
# multiplication
print(x * y)
# Division
print(x / y)
# Modulus
print(x % y)
# Exponentiation
print(x ** y)
Comparison operators that return True or False values:
# less than
print(x < y)
# less than or equals
print(x <= y)
# greater than
print(x > y)
# greater than or equals
print(x >= y)
# equals
print(x == y)
# not equals
print(x != y)
Logical operators are used to combine comparison operators. The and operator return True if both conditions are True. The or operator returns True if at least one of the conditions is True. The not operator reverses the boolean value of the condition:
# and
print(x > 5 and y <= 3)
# or
print(x < 20 or y < 5)
# not
print(not (x == 10))
You may have noticed the hash (#) symbol in our code. This is how single-line comments are written in Python. Any text following the # symbol is ignored by the interpreter, allowing you to include notes or explanations in your code.
Control Flow in Python
Control flow structures are crucial in programming because they help determine the order in which the program’s instructions are executed. Python provides an easy and clear way to manage this flow using conditional statements and loops,
Conditional statements execute specific blocks of code based on certain conditions. In Python, the if, elif, and else keywords are used for decision-making. First, Python evaluates the if statement. If it returns False, the program then evaluates any elif statements. If all if and elif conditions are False, the program executes the code in the else block. If the condition in the if statement or any elif statement evaluates to True, it runs the corresponding block of code immediately:
score = 85
if score >= 90:
print("Grade: A")
elif score >= 75:
print("Grade: B")
elif score >= 50:
print("Grade: C")
else:
print("Grade: F")
Loops allow you to execute a block of code repeatedly. Python supports two types of loops: for and while. The for loop is used to iterate over a sequence like a list, tuple, or range.
# iterating over a list
fruits = ["apple", "banana", "cherry"]
for fruit in fruits:
print(fruit)
# iterating with range
for i in range(1, 10):
print(i)
The while loop continues as long as the condition remains True.
# The while loop
count = 5
while count > 0:
print(count)
count -= 1
When working with while loops, it is important to be cautious and ensure that the loop has a condition that will eventually become False. If the condition never evaluates to False, the loop will run indefinitely, creating an infinite loop. In the example, notice how the value of the count variable decreases with each iteration. The loop continues executing as long as the condition count > 0 remains True. Once the count reaches 0, the condition evaluates to False, and the loop stops executing. This illustrates how modifying variables within the loop helps guide the condition toward becoming False, ultimately terminating the loop. It’s essential to always plan for a clear exit condition to prevent unintended infinite loops.
Functions in Python
Functions are reusable blocks of code that are designed to perform specific tasks. They help improve the organization, modularity, and maintainability of your programs. A function consists of a set of instructions grouped under a name, which can be called whenever needed. Python provides built-in functions such as print() and input(), but you can also define your own functions, known as user-defined functions, to suit your specific needs.
To define a function in Python, use the def keyword, followed by the function name, parentheses, and a colon. The function body is indented:
# function without a parameter and return value
def greet():
print("Hello, World!")
# calling the function
greet()
Functions can have parameters. Parameters are variables listed in the function definition. Arguments are the values passed to the function when it is called:
# function with the parameter name but no return value
def greet_user(name):
print(f"Hello, {name}!")
# calling the function with the argument Doe
greet_user("Doe")
The return statement allows a function to send data back to the caller. Without return, a function returns None:
def add_numbers(a, b):
return a + b
result = add_numbers(5, 3)
print(result)
Installing and Using Packages
Python's versatility lies in its ability to extend functionality with the help of packages. These are pre-written code libraries that allow developers to build complex applications quickly and efficiently. Whether you are developing simple scripts or large-scale applications, you will often need to install and manage packages. You can install packages using pip or with a requirements.txt file.
pip
is Python's package manager, and it allows you to install, upgrade, or remove Python packages. You can use it directly from the command line or terminal:
# install the latest version of a package
pip install package_name
# install a particular version of a package
pip install package_name==version
# uninstall a package
pip uninstall package_name
With the requirements.txt file, you list the packages:
package_name1
package_name2
package_name3
You can install specific version of the packages:
package_name1==version
package_name2==version
package_name3==version
You can then install the packages in the requirement.txt file with pip:
pip install -r requirements.txt
You can get details about an installed package with:
pip show package_name
Let’s demonstrate how this works by installing the numpy and matplotlib packages and make a simple plot. First, list the packages in a requirements.txt file in the root directory and install the packages with pip:
numpy
matplotlib
You can confirm that the packages have been installed in the virtual environment with pip freeze or pip show:

Next, create a plot.py file with the following code and run:
import numpy as np
import matplotlib
matplotlib.use('Agg')
import matplotlib.pyplot as plt
# Generate data for a sine wave
x = np.linspace(0, 10, 500)
y = np.sin(x)
# Plot the sine wave
plt.plot(x, y, label="Sine Wave", color="blue")
plt.title("Sine Wave")
plt.xlabel("x-axis")
plt.ylabel("y-axis")
plt.legend()
plt.grid(True)
plt.savefig('plot.png')
A file named plot.png is saved in the root directory:

Conclusion
Python is an excellent choice for beginners starting their programming journey due to its simplicity, readability, and versatility. This tutorial has guided you through setting up Python and its development environment, understanding basic syntax and data types, and exploring essential programming concepts like control flow, functions, and package management. With practical examples and tools like PyCharm, you are now equipped to write, debug, and execute Python programs confidently. As you continue to learn, Python's expansive ecosystem and user-friendly design will empower you to tackle projects ranging from data analysis to web development and beyond.
like this