Improve Your Code with Loops in Kotlin
Imagine having to repeatedly write a bunch of elements or repeat something, like memorizing multiplication tables in elementary school. Did you have to write out the entire table with repeated multiplication statements?
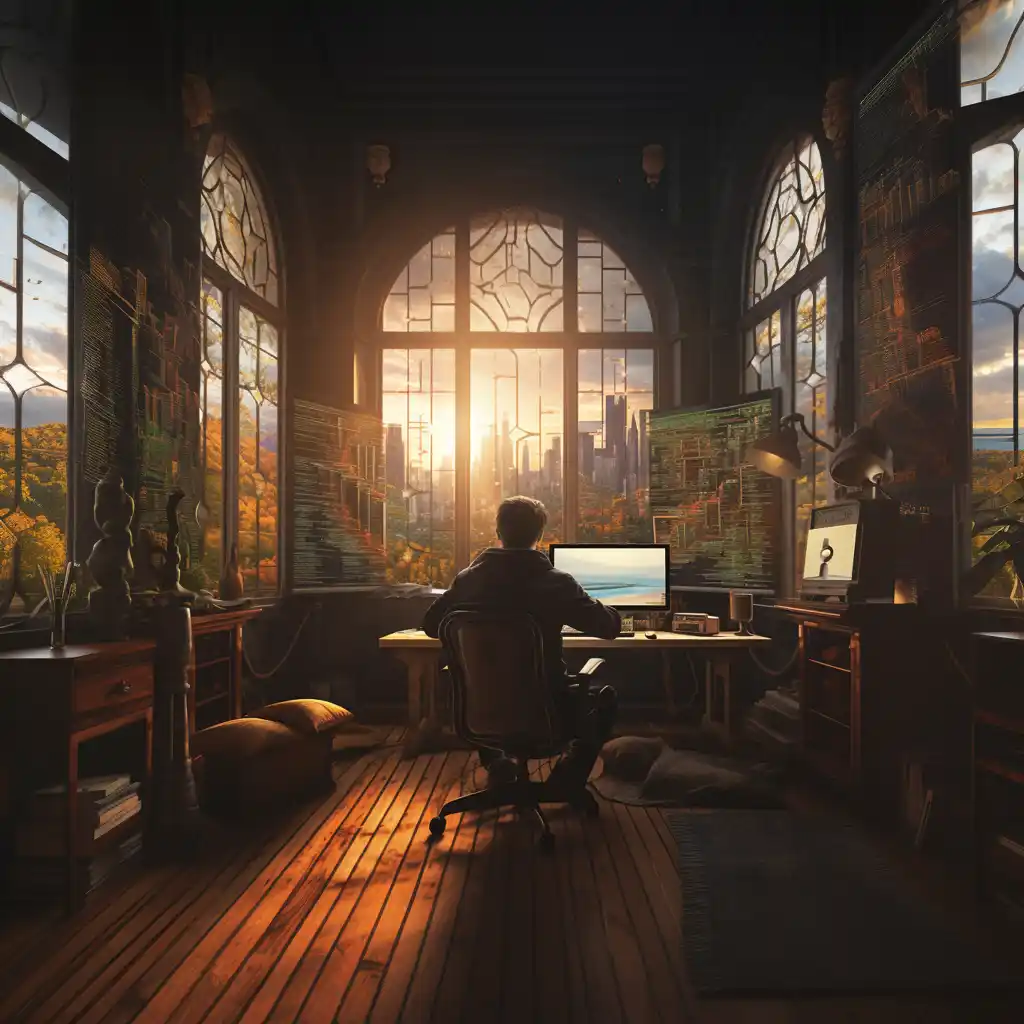
If we need to write the multiplication table, we will write something like this:
You can try the code in the link below or see it below. The code is the same.
Step one - identify the problem
As you can see, the whole thing becomes too lengthy, and this is only the part of the multiplication table with the digit one. What if you need to write the entire multiplication table? How would you write it?
Here is the whole multiplication table:
(You can find the code on the link below or in the area below the link. The code is identical.)
That is written in 100 lines.
This is something that is a lot of work, isn’t it?
Step two - turn your question into a solution
Fortunately, every programming language provides us with a tool called a loop.
In Kotlin, we have four types of loops: for, while, do while, and repeat.
Programmers consider the for loop to be the most flexible. Most often, the for loop has such a structure:
You can try the code on the link below or see it in the area below the link. The code matches.
The for loop can be visualized as a rotating circle, with each rotation being called an iteration. We can attach a logic to this circle and repeat it as many times as we need. The for loop has a pointer that points to each iteration, with the constant "i" representing the current iteration number. Using the for loop, we can quickly write the multiplication table and perform other repetitive tasks efficiently.
Check this example code:
You can try the code on the link below or see it in the area below the link. The code matches.
In this example code, we use two nested loops. The external one calculates the first multiplier—1, 2, 3, etc. and the internal one calculates the second multiplier, whose value repeats from 1 to 10 for every value in the external loop.
As you can see, 100 lines of code become just seven if we don’t count the primary method. In Kotlin, the most often used form of for loop expects a range object that can represent closed ranges: “...”
1...4 will represent numbers from 1 to 4. This example loop will print the numbers from 1 to 4:
You can try the code on the link below or see it in the area below the link. The code matches.
or open ranged – e.g., 1..<4.
This example loop will print individual numbers from 1 to 3:
You can try the code on the link below or see it in the area below the link. The code matches.
Step three - use the range expression
Since the range in Kotlin is potent, we can do many things with it.
For instance, we can count backward by using the downTo method of range object:
You can try the sequence of code on the link below, or see it in the area below the link. The code is the same.
We can use iterator with steps. This example code will iterate with step 3 items through our range:
You can try the code on the link below or see it in the area below the link. There is no difference.
Loops support two essential things. Break and continue keywords. With them, you can skip iteration or break the current loop completely.
This example will return only one because all other iterations will be skipped.
You can try the code on the link below or see it in the area below the link. The code matches.
In the example below, nothing will be printed because the break keyword interrupts the loop immediately.
You can try the code on the link below or see it in the area below the link. The code is the same.
As a high-end language, Kotlin supports other valuable loops. For instance, a repeat loop is sound when you want to do something several times and know how many times you want to do it.
You can try the code on the link below or see it in the area below the link. The code is the same.
Master the classic Kotlin loops
There are also a couple of classic loops that Kotlin supports too.
The while loop is a loop where a block of code will repeat until a Boolean expression becomes false. We use this type of loop when we need to perform a task, but we are still determining the number of times we will need to repeat it. For instance, imagine a sink full of dirty dishes. You will keep washing the dishes as long as there are dishes in the sink, right?
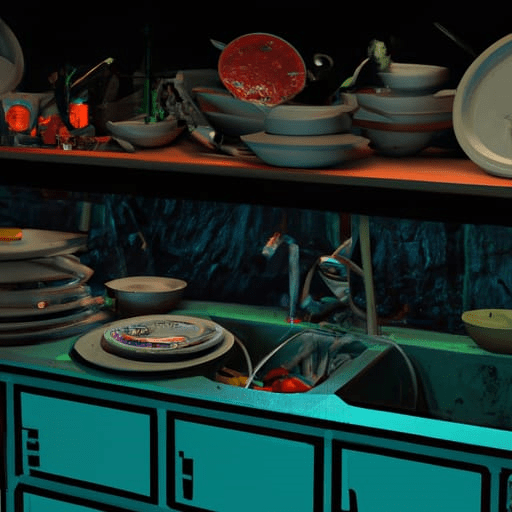
Here is an example of the while loop. In this case, we will repeat to print the first hero in the list until there are heroes. It will print Superman, Wonder Woman, and Batman. Every hero is on a new line.
You can try the code on the link below or see it in the area below the link. The code is the same.
The other classic loop that Kotlin supports is a do while loop. It consists of the following structure:
The loop begins by executing the do loop code block, executed every time, regardless of whether the condition is true or false. After the first iteration, the conditional expression inside the while brackets is evaluated, and the loop continues to the next iteration only if it is evaluated to be true. This evaluation occurs at the end of each iteration inside the do block.
You can check this example code:
This code accepts and prints the range of values until the user types stop.
This is the output of this program:
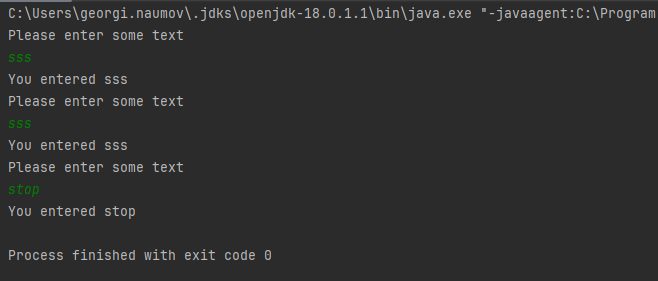
Conclusion
We have covered the topic of loops in Kotlin. I hope you have enjoyed our journey so far and feel your performance being boosted. To wrap things up, we will provide an example application that can assist someone in studying the multiplication table. This application will test the user on their knowledge of the multiplication table, and if they succeed, it will print the message Congratulations! You know the multiplication table!
like this