Getting the Most from Keywords in Python
In this article, we will discuss keywords and soft keywords in Python: what they are and how to use them. I will introduce you to all the keywords and soft keywords in Python, providing necessary examples.
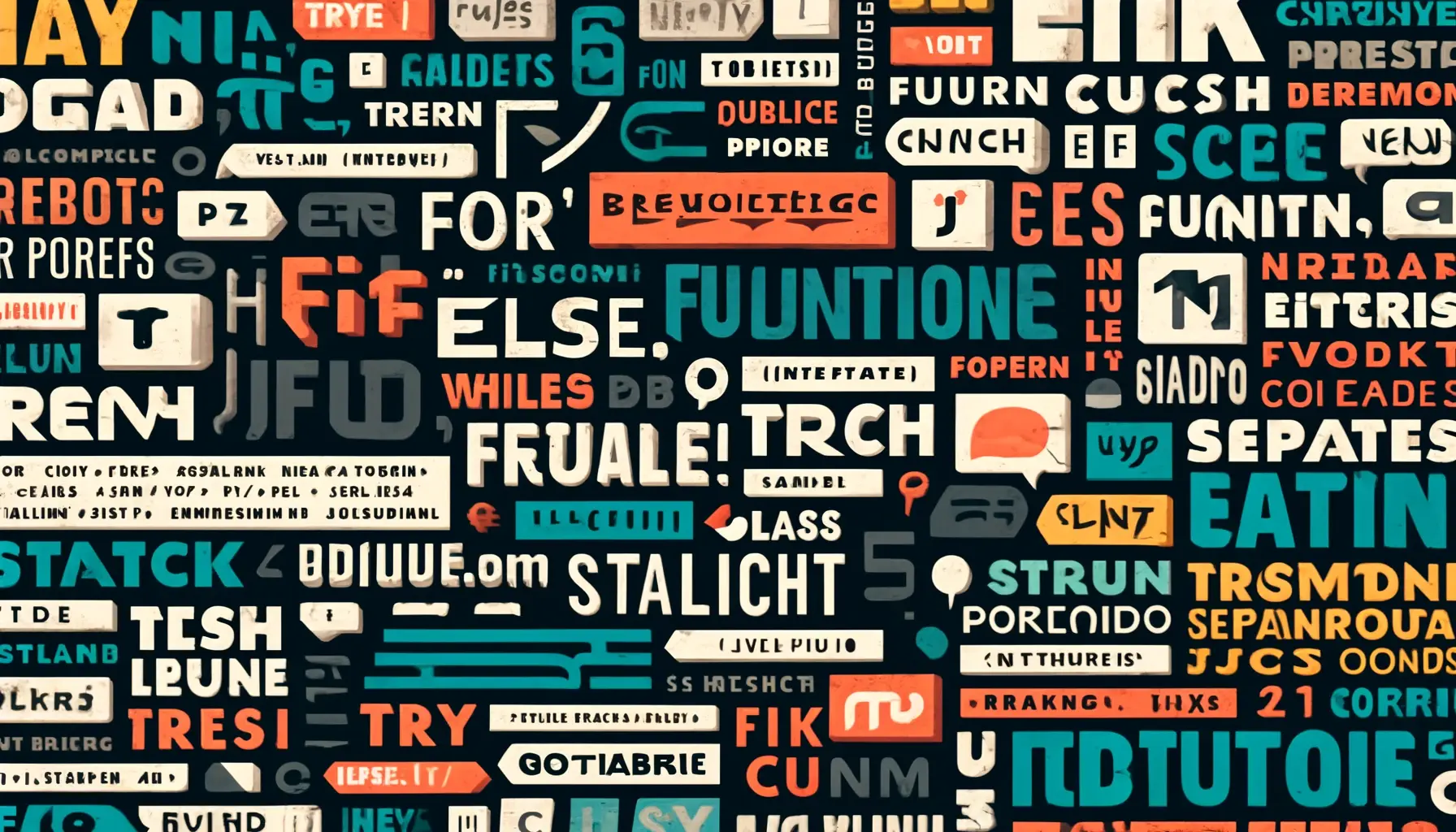
What are keywords?
Keywords are special words reserved for a specific purpose, and they cannot be used for anything else. For example, def is used when constructing a function. If you try to use this word as a name (identifier, function name, variable name) in your code, you will get a SyntaxError. This means that you attempted an action that is not allowed, and Python prevented you from doing so. The same applies to all keywords. A small exception exists for the words None, True, and False. While you still can't use them as names, you can at least print them! π We will learn why later.
β
What about built-in functions like str or bin? Are these keywords? No, they are not. The list of keywords is well-defined, and we will examine it shortly. Unfortunately, since str is not a keyword, all Pythonistas are destined to encounter str = 'hello π' in a beginner's code. π±
Note: It's worth mentioning that you also can't reassign keywords to other variables. For example, you cannot use T as a keyword instead of class (T = class).
Note: The term 'keyword argument' has nothing to do with keywords. For instance, in complex(real=9, imag=0), real=9 and imag=0 are keyword arguments, meaning that an argument is preceded by an identifier.
Motivation
Knowing the keywords of your language is crucial. They are the building blocks that help you make your dream app a reality. Keywords define the entire flow of your program. Any concept you use or idea you try to implement is related to keywords. Potentially, you could write a program without a keyword, such as a quine c = 'c = %r; print(c %% c)'; print(c % c), but is this the peak of your imagination?
Without keywords, you cannot make your program object-oriented, handle exceptions, control your flow and input, define functions to ease the work, write asynchronous code, use antigravity, and much more.
β
To fully enjoy and master the language, you must be familiar with its keywords. So, are you ready to explore the keywords that all Pythonistas use every day? If yes, then suit up, and let's go.
β
Keywords
Python has 35 keywords. For ease of understanding, we can divide all keywords into a few subjective subcategories.
All keywords are case-sensitive, so if the given keyword is True, it works only as True and not as true. Keep this in mind while applying keywords to your code. You can also get a list of all keywords at runtime, ensuring no keyword is overlooked. To view them as a Python list, you can do the following:
For completeness, add keyword.softkwlist to see the soft keywords.
Keywords output:
Soft Keywords output:
Boolean Manipulations: True, False, not, or, and
The keywords True and False represent boolean values. Many programming languages have human-readable names for these values, and Python is no exception. It's clear that they represent the truth of an expression. Notice that both words are capitalized. Since Python is a case-sensitive language, you must write them exactly as they are. Otherwise, it won't work. One key difference from other keywords (not including None) is that these values are constants. This means that you can use them directly in your code. Let's try it:
β
The first line prints True, indicating that the first expression is true, and the second line prints False, indicating that it's not true.
β
While writing them by themselves might seem pointless, they represent a fundamental concept that you will use with more complex expressions. Let's look at some examples:
The first one evaluates to False, since 1 is not strictly larger than 2, and the second one evaluates to True, since 2 is indeed strictly larger than 1. In both these examples, you see True or False values as your output even if you didn't specify them explicitly.
β
Simple expressions are not as much fun to look at, so let's try another example and see what it will evaluate to.
Do you know the result? The result is True.
β
0 < 1 is True
12 + True > 12 is True
{} is False π
β
So, it's True and True or False and it's time to talk about what's going on here in more detail.
β
and and or represent the boolean operators. They allow you to work with more complex boolean expressions, such that the result depends on multiple criteria.
β
and evaluates to true if and only if both the left and the right parts of it are true. For example, True and True evaluates to True, True and False evaluates to False, False and True evaluates to False.
β
or evaluates to true if at least one side of or is true. True or True evaluates to True, True or False evaluates to True, False or True evaluates to True, and False or False evaluates to False.
β
Note that the chain of execution follows the short-circuit evaluation. In practice, this means that when the minimum condition in the chain is satisfied, the rest of the chain's evaluation is not necessary, so it stops. For example, True or True or True or True or False or β¦. After the first True, it stops and returns True because it understands that no additional calculations are necessary if one side of the or is True.
β
Here is one more example: (True or False) and False. In this example, True or False is evaluated first, without evaluating the second operand of the or operator (because it knows that only one part for the or should be true). Next, it evaluates both operands of True and False expression that results in False.
β
not is nothing else but the negation of the given boolean value.
β
Even if the concept of True/False and operations on them is simple, Python has a few quirks that one might want to know about.
First, you can use True as the value 1, and False as the value 0. For example, if you need to count the number of expressions that are evaluated to True, you can simply do this (it is a toy example, don't bite me):
And yes, you can add them to any other number. 1 + True yields 2. 1 / False raises a ZeroDivisionError π
The next behavior that you might not expect is the result of something like this:
β
This prints {1}. The reason is that Python returns the last evaluated part of an expression. While it might look like something awful, in practice it's quite handy. For example:
β
In this case, some_array in a boolean context will be evaluated to True if it has any elements in it. So, no additional checks are needed to stop the while loop.
β
In this case, if get_ip() returns None, it's quite handy to set some default value with the help of the or operator.
β
As a last bit, let's say what values are False when they're treated in a boolean context. As the docs say, the values are - False, None, numeric zero of all types, empty strings and containers (including strings, tuples, lists, dictionaries, sets, and frozen sets). Anything else will be evaluated as True. You can check what is what by doing bool(...).
β
Pro tip: Since boolean expressions evaluate to a boolean, return the expression instead of doing something like print(True if 'some boolean result' else False). Don't forget that if any other object is involved, it may return that object instead of the plain False/True value.
β
Pro tip: Use β¦ is True / β¦ is False to check for truthiness explicitly if necessary.
The None Keyword
None is used to represent the absence of a value. Nothing else is like None. It's also returned by default from any function if the return statement is not used. Note that, as with True/False, None is also a capitalized word and a constant. However, even if bool(None) is False, you can't add them together or to anything else (this results in a TypeError). Let's look at some examples:
β
Here, the function foo doesn't return anything explicitly, and by default, the value None is returned.
The value arr is also set to a default None. In this case, it's recommended to use None, since a list is mutable and may lead to unpredictable results. For example, if you use recursion and want to feed a mutated array for a recursive call.
β
Pro Tip: As with True/False, it's recommended to use β¦ is None when you need to check if some value is None. For example:
β
In this case, if we pass the value 0 to the function, it won't confuse it with None and will not print that itβs just a number.
β
For now, you might have recognized some other keywords that we didn't cover. Don't worry, we will talk about them sooner or later.
Conditional Statements: if, elif, else
We've seen some of these at work, but what exactly do they do? These statements are part of the so-called "control flow" of the program. The if and elif statements accept a condition (that can be evaluated as True or False) and execute the first block of code that meets this condition. If no conditions are met, then the else block is executed. The elif and else statements are optional; you can use a single if, if/else, if/elif, if/elif/elif/... or if/elif/else.Β
Let's look at an example:
β
In this example, we compare age with a value, which evaluates to True or False. In this case, both age < 15 and age < 25 are False. So we go to the next condition, age < 50, which evaluates to True, so we print You're counting your age in decades. If there were more conditions, or if the first one was evaluated to True, the rest wouldn't be evaluated and it would continue at the next line after the else block.
β
There's nothing particularly difficult about this, just make sure the syntax is correct (the keyword, a boolean expression, and a colon ":" at the end of the expression) and that all expressions follow logically. For example:
β
Perhaps you intended to print 'Howdy mate!', but because of the flawed logic, you get 'Oh, hello there.' and age > 30 will never be evaluated.
β
Note: if/elif/else has a one-line shortcut idiom:
β
This is okay to use with short expressions, but it can be quite challenging to read longer ones.
β
It's important to note that you need to keep your if blocks connected. For example:
is not the same as
Can you guess why?
Time to Fly: from, import, as
It's not a secret that you can "fly" with Python, as humorously depicted in this XKCD comic.
But what does the import antigravity statement in that comic do? Try it yourself and find out!
β
The import statement is used to add different external or local modules to your program. This allows you to enhance the functionality of your application without having to implement any complex algorithms yourself. Let's look at some examples.
β
Have you ever come across the math module? If not, you can simply do the following:
β
This will import the math module, and you can use its constants and methods from it. It is as simple and easy as it looks.
The import system also supports importing single functions and constants with the help of the from keyword.
β
If you don't like the imported name, you can use another keyword as to help you with aliases.
β
Let's enhance this humble description with some best practices, shall we?
- As demonstrated above, you can import names with your aliases. This helps to avoid name collisions. Remember to set descriptive and meaningful names!
- Avoid wildcard imports! Polluting the namespace with tons of unused functions is never good for your code.
- Follow the PEP 8 recommendations to organize your imports.
Be the Exception: raise, try, except, finally, assert
Python's exception system is a robust mechanism that helps manage all types of errors that your code might encounter. It ensures that you have full control over everything you write, providing stability, and reliability, and it's a significant time saver. Here's an example:
β
In this example, we're using the exception-handling mechanism to its fullest. The try block attempts to open a file named 'example.txt'. If the file doesn't exist, it will throw an exception. The FileNotFoundError is caught in the except statement. Note that you are given a handy alias fnf_error for the exception. You can extract information from the fnf_error object and take control of it. In this case, we're just printing the object. If any other exception occurs, we will catch it with the except Exception as e block. Here, we also have full control over the exception. The else block is something that you might not expect to see. The else block is executed if no exceptions occur. Finally, the finally block! This block is always executed. If you have some unfinished business, or if you want to clean up your code, feel free to use the finally block.
β
Note that else and finally blocks are optional; you can use both of them, only one of them, or none at all.
Another possibility is to use try/finally pair. Let's look at an example that demonstrates that the finally block always executes:
β
What do you think is the result of the function? At first glance, you might think that it prints True or even None. That's a reasonable guess. However, remember that finally always executes! And it executes return False, so it prints False. It might look counterintuitive at first, but you need to understand that, unless there are some extraordinary circumstances, the finally block is always the last to execute!
β
Exception handling has a newer syntax that allows you to handle multiple exceptions in groups. Let's look at an example from the documentation:
This is the output:
You can refer to the official documentation for a detailed explanation; this example serves as a syntax demonstration with the try/except keywords.
β
Pro tip: try/except is a time saver. At first, it feels wrong when you do it, but handling some data validation inside of the try/except is a common Python practice and an idiom. Don't be shy, save your time with a try/except block.
β
Another keyword that fits perfectly in this subsection is the raise statement. You might already guessed what it does - it raises exceptions! Whenever you encounter a situation where you feel it's appropriate to raise an exception, you should do so. Here's a simple example:
β
In this case, you're implicitly raising the Exception. You can handle it right away, but is there a point in doing so?
As you can see, the syntax for this is simple. First, you use raise, then you specify the class of the Exception, and finally, you specify the message to the user. You can also use the single raise statement. It's used to re-raise the previous exception. If no exception occurs, you will get RuntimeError: No active exception to re-raise.
β
Last but not least is the assert statement:
β
The assert statement allows you to check if conditions evaluate to True at some crucial point in your code. assert statements are useful for debugging, and you should never use them in production code.
The syntax for that is as follows: first, the assert keyword, then the boolean expression. If the expression evaluates to False, it throws an AssertionError. The useful message is optional, and you can avoid it if you know what you're doing. Note, that as this is a boolean, you can mix any complex chain as you desire.
Create and control the loop: for, while, continue, break
The for loop is a crucial building block of the Python language. With a for loop, you can iterate over any sequence (lists, tuples, strings, ranges) or anything iterable.
Many languages have a for loop. The classic example of the for loop is something like this:
β
However, Python is not Python without cool syntactic sugar. As you remember, you can iterate over any iterable, so why not just iterate over a list?
β
If you want to keep counting, you can always enhance it with an enumerate function.
β
You can always break out of the loop with a break statement. Let's say your mission is to find an element in an array, and you don't want to waste CPU power on iterating the whole array if you've already found the magic element.
β
In this case, you will see numbers from 0 to 5 (inclusive) printed on your screen.
If you want, you can add an else clause! What? With the loop? You might ask. Yes! With a loop! The else clause is executed only if the whole iterable is exhausted. For example:
β
Since we went through all elements of an iterable, the message 'Hello' will be printed at the end. However, if you break and quit the loop before it's exhausted, no message will be printed.
The continue statement is used in the loop if you want to skip some specific position or element or for any other reason you might have in mind.
β
In this case, the number 5 is not going to be printed.
The while loop is similar to the for loop, with the exception that it stops if the provided boolean expression is not true.
β
Here's the output:
β
The else statement works similarly with while loops. The else clause is triggered if the loop exits because the boolean expression is False.
The pass statement
The pass statement is one of the simplest keywords; it acts as a no-op and can be added to any block of code. You can use it as a placeholder if you want to finish something later.
β
Note: The pass statement is a no-op operation. It doesn't act as break, continue, or return. After the interpreter reads it, it continues to read the next line, as if nothing happened. Indeed, nothing happened!
Working with functions: def, return, yield, lambda
Let's explore the world of functions a bit closer. We've seen some previous examples that used some of these words, for example, def. The def keyword is the syntax to define a function in your code. It serves this purpose and nothing else. To define a function, just write def followed by the name of the function, parentheses (zero or more arguments), and a colon. Donβt forget to pay attention to the indentation inside of the function body.
You might want to use pass if you don't know what the function is for and would like to come back to it later.
β
The function is quite useless at the moment, so let's make another one that adds two numbers.
β
As you can see, we use the return statement to return the result of our mathematical expression.
In this example, return is the last statement that is processed in the function; nothing after that matters.
β
In such an example, the third return is unnecessary, since else is executed no matter what.
It may make a lot of sense to define your function like this instead:
β
Another way to return a value from the function is to use yield (yield from).
β
Yield creates a generator from the function that makes it possible to retrieve a single value at a time.
The last thing about functions is that you can use the lambda keyword to create anonymous functions (the possibility to bind it to a name still exists tho).
If we repeat print statements from the previous example, we will get the same result. Of course, this is not a definitive guide on functions, so if you want to learn more about it please refer to documentation or some external resources.
The class keyword
As you might have guessed, the keyword class is used to create your custom objects and enhance your code with an object-oriented style.
β
Here you go, using the keyword class and a name, we created a custom class for creating our objects. Unfortunately, this class does nothing. In some circumstances, you might need it, but let's enhance it just for now:
β
As you can see, a single keyword opens the door to a whole new paradigm. To learn more about classes, you might refer to this documentation.
The world of asynchronous programming: async/await
These keywords are used for handling asynchronous programming, which is the concurrent execution of different subroutines. It may lead to increased performance, more efficient use of resources, etc. Here is a simple example:
β
The async keyword is used to declare a function as a coroutine (you can learn more about it here), which makes it a special kind of function that you can't simply invoke. In this example, I use asyncio.run to invoke the function.
The await keyword is used inside an async function and waits until the completion of any awaitable object. In this example, we use the awaitable sleep method from asyncio and pause the function execution for 1 second. After that, the function continues its execution.
Choose your scope: global, nonlocal
These two keywords are used as helpers to decide which names to refer to in the current scope. Let's look at a simple example:
β
β
In this example, we have three variables named x, inside the inner function, we use nonlocal x to set the scope to a non-global scope. That's any scope 'above' the local and not global is treated as nonlocal. Hence the output, the global x is the same, and the non-global x has changed.
If you change nonlocal x to global x, then the opposite will happen; the only x that will be changed is global, and the non-global x (inside the outer function) will stay the same.
The mighty four: del, in, is, with
Ok, last but not least, the mighty four keywords that I couldn't group with any other. π
The del statement is used to delete objects. For example:
β
As you can see, it removes the whole variable, not just nullifies the value.
β
In this example, we remove the value at the 2nd index of the array x.
Probably one of the most useful examples is removing a key from a dictionary.
β
The in operator checks if the target value is in some collection. For example:
β
Output:
β
As you can see, you can easily test for membership without writing a custom algorithm for that.
Quick quiz, what do you think? Is it a fast operation to check if some number is in the range or not, and why?
Test your intuition with this simple snippet
β
The is operator tests for the objects' identity. It's equivalent to id(obj1) == id(obj2).
The preferred usage for is is with the None constant, as shown above in the part about None.
If you want to compare two values, use == instead of is.
β
One may say that the with statement creates a context manager. That is, it delegates some functionality to a manager. It ensures that resources are acquired safely before entering the block and released reliably afterward, even if exceptions occur. One of the classic examples is reading a file. The old way is something like this:
β
In this example, we explicitly closed the file. However, with a context manager, files are closed automatically. It's also more readable and pleasant.
β
To be able to use this with your custom classes, you need to define __enter__() and __exit__() magic methods for your class!
β
That's it for the main keywords. Next on the menu are soft keywords.
β
Soft keywords
In Python, soft keywords are something even more special. If you reassign them, they don't throw an exception as with regular keywords. Moreover, in daily life, most Python programmers use one of these as a name for their variable. So, let's begin.
The soft keyword that is often used as a throwaway variable is _. If you don't want to use some variable, you set the name as _.
What makes it a soft keyword? The answer to this is context. In Python 3.10, Python introduced structural pattern matching, where three soft keywords are used. Let's take an example and try to explain what each one is doing.
The match statement
Here we see three of the keywords in use. It works similarly to Rust's pattern matching. The match statement operates on the matching subject (in this case it is status), trying to match it with one of the given cases. Based on the provided input, it should match with the correct block. If none are matched, the wildcard (_) block is executed. The whole topic is much more interesting and contains a handful of tools for your coding journey, so I recommend getting familiar with it.
Type statement
The last one, probably not that well-known at the moment, is the type statement. Attention: not the function, the statement. Here is an example:
β
This is simply type aliases for more complex structures. It allows you to define a name before it appears in code. As you can see, it supports generic types as well. Remember value: int = 2? Well, now you can do point: Point before you even define this class!
If you want to learn more about type hints please refer to this pep.
β
Wrap up
I hope you've gotten a hang of keywords and soft keywords. I tried to elaborate more on the ones that people make more mistakes with, and provided a shorter description for simpler or newer keywords. As no single article is complete (and no one wants to read 37 articles in one π), I recommend you go deeper with a keyword that you're most interested in and learn more about it. Have you applied type aliases in your code yet? What about the match statement? It looks very promising.
Good luck on your coding journey and using new keywords in your code!
β
like this