Fixing Objects Not Valid as React Child Error
The Objects are not valid as a React child error in React typically occurs when developers try to render an object directly in JSX.
In React, JSX can render text, numbers, valid React elements, or arrays of these types, but not objects directly.
This error often happens in the following scenarios.

Rendering an Object Directly
You'll encounter this error if you try to use the render method for a plain JavaScript object in JSX.
For instance, if you have a custom component:

The user is an object, and React doesn't know how to display this object as it's not a valid JSX element.
Incorrect Data Structure
You might unintentionally pass an object instead of a string or number.
This can happen if you're fetching data from an API and not correctly handling the data structure before rendering it on your application.
Imagine you have a React child component that fetches user data from an API and tries to display the user interface. However, instead of typical behavior showing a specific property of the user object, the code attempts to render the entire object, leading to an error message.
Here's a simplified example:
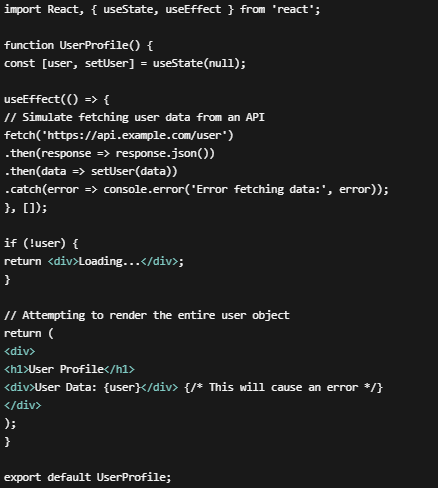
In this example, the <div>User Data: {user}</div> line is problematic. A JavaScript object represents the data fetched from the API. React cannot render JavaScript objects directly into the DOM.
The solution to this issue is to access specific properties of the user object. For example, if the app component has properties like name and email, you should display these properties individually:
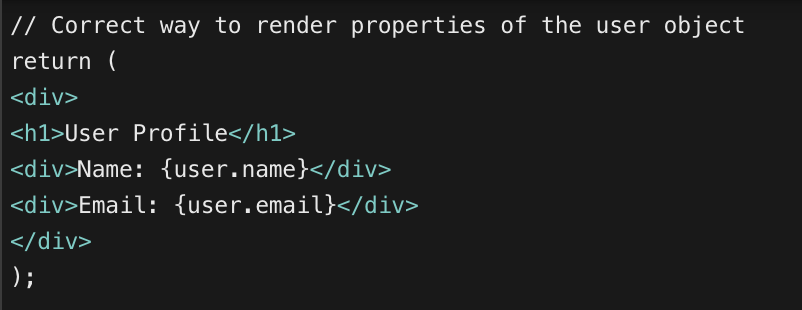
This approach ensures you render valid JSX content (strings, numbers, or React elements), not entire objects.
Nested Objects in Arrays
If you render an array using .map() or similar built-in array methods, and the elements of the array are objects, you'll get this standard error. Each element in the array of elements must be a valid JSX element or a text/number.
Imagine a React component that displays a list of users. Each user is represented as an object in an array of objects. If you try to render this array of objects directly, React will throw an error because it can't render objects as JSX elements.
Here's an example that illustrates this problem:

In this example, the {users.map(user => <li key={user.id}>{user}</li>)} line attempts to render each user object inside a list item (<li>). This is invalid because the user is an object, and JSX can only render strings, numbers, React elements, or fragments.
To fix this, you should use the technique of rendering specific properties of each object:
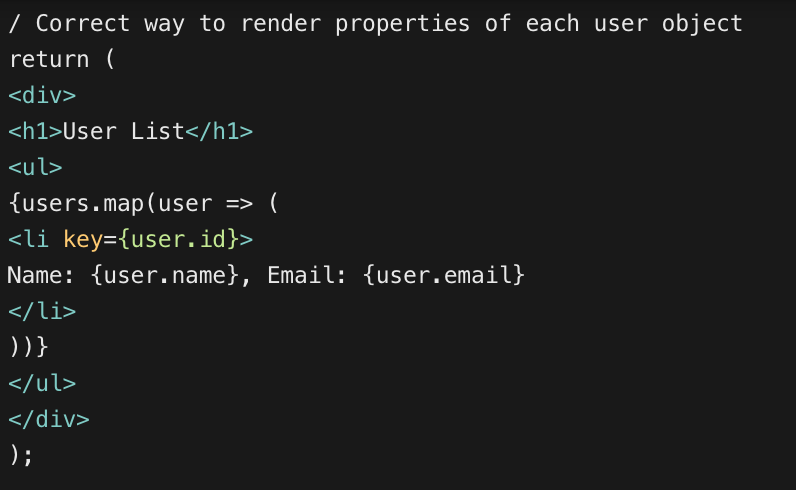
In this corrected version, each list item displays the name and email properties of the user object, which are valid types for rendering in JSX. This approach avoids the error and correctly displays the user information.
like this
Conclusion
To fix this error, you should:
- Convert Objects to Strings: If you need to display an object, consider converting it to a string first, using JSON.stringify(object) for debugging purposes, especially complex objects.
- Render Specific Object Properties: Instead of rendering the whole object, render specific properties. For example, instead of <div>{user}</div>, use <div>{user.name}</div> to display the user's name.
- Ensure Proper Data Structure: Ensure the data you're trying to render is in the correct format. You'll need to adjust your data handling logic if it's an object when it should be a string or number.
Correctly handling data types in JSX is crucial to avoid errors in React. Refer to React docs and technical documentation for more information.
Something Extra
Using TypeScript in a React project can significantly reduce the likelihood of encountering the Objects are not valid as a React child error, thanks to its static type-checking features.
TypeScript enforces type safety, catching mistakes like rendering objects directly in JSX before runtime.
Here's how TypeScript can help in the scenarios mentioned:
Type Annotations for Parent Component State and Props: In TypeScript, you can define the types of children's props and states in your components. If you have a component that expects to render user data, you can specify that the user data should be an object with specific properties. TypeScript will then enforce that you use these properties correctly.
For example:

Enforcing Correct Data Structures When Fetching Data: When fetching data from an API, TypeScript can enforce the expected structure of the response. This ensures you handle the data correctly before setting it to your component's state.
Example:
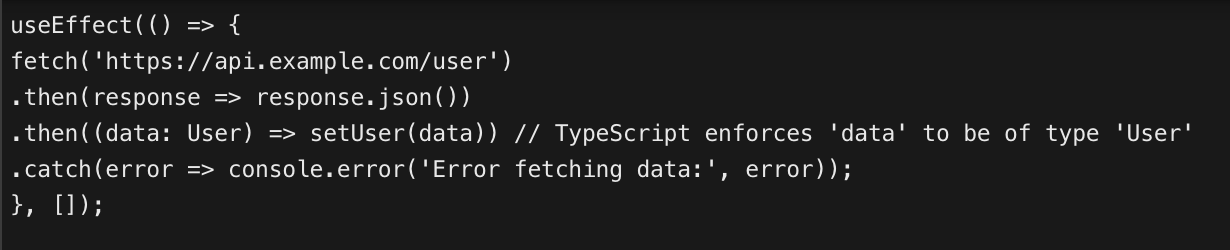
Array Rendering with TypeScript: TypeScript can enforce that arrays used in rendering contain elements of a specific type, helping you avoid trying to render objects directly.
For instance:
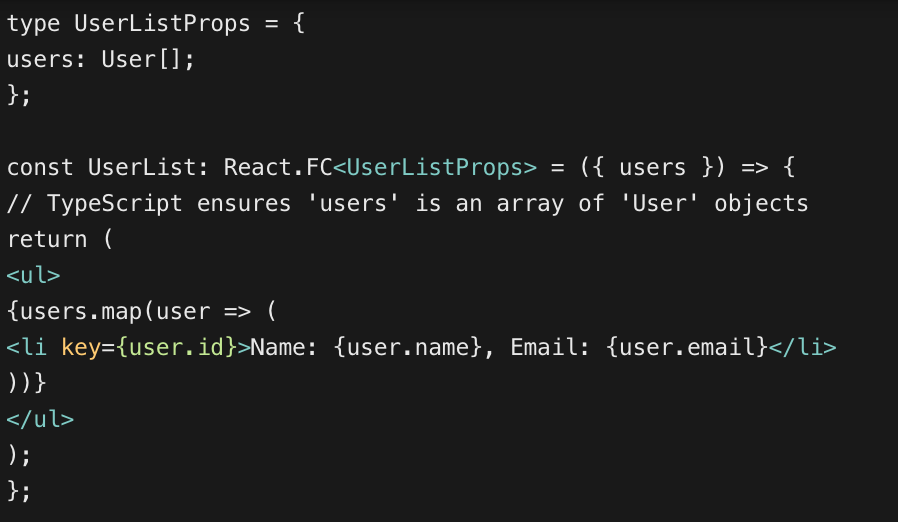
TypeScript acts as a safety net in all these cases by catching type-related errors during development time before the code is compiled and run. This not only helps prevent the specific error of rendering objects directly in JSX but also improves the quality and maintainability of the code between components.