Enhance Code Readability with <StrictMode>
According to the React documentation, <StrictMode> lets you find common bugs in your components early during development. Thus, <StrictMode> is used to identify and fix potential errors in the application. It can be applied to the entire application or specific components and parts of the application. Since it is a developer tool, it is used in development mode. <StrictMode> renders each component twice to identify any issues in the application and shows warning messages in the console.
.webp)
The Importance of Code Readability
Code readability plays a key role in software development. Clear and clean code makes it easier to maintain and develop projects, reduces the time needed to find and fix bugs, and improves teamwork.
Advantages of <StrictMode> for Code Readability
The advantages of <StrictMode> are numerous and significant.
Firstly, Strict Mode prevents silent errors by turning them into exceptions, making code debugging easier.
Secondly, it prohibits the use of outdated syntax and potentially dangerous constructs, such as accidentally created global variables.
Examples of advantages:
- Safety: Strict Mode forbids certain unsafe actions, like assigning values to undeclared variables.
- Performance: Certain code optimizations can only be performed in Strict Mode because it provides additional information about the code to the JavaScript engine.
- Clarity: Code becomes more understandable and predictable because Strict Mode reduces the number of possible “magic” behaviors.
<StrictMode> makes errors more apparent and simplifies debugging, making it an invaluable tool for modern development. Transitioning to <StrictMode> is a step forward towards writing clean, efficient, and secure code.
Now, let's look at examples of how <StrictMode> works.
Examples of Using <StrictMode>
To enable Strict Mode for the entire application, wrap the root component with <StrictMode> during its rendering:
You can also enable <StrictMode> for any part of your application:
Fixing Errors Caused by Double Rendering During Development
React requires each component to be a pure function that returns the same JSX given the same inputs (props, state, context). If a component is not pure, it behaves unpredictably and causes errors. Strict Mode helps identify such errors by invoking some functions twice during development:
- The main logic of a component (excluding event handlers)
- Functions passed to useState, set functions, useMemo, or useReducer
- Certain class component methods, like constructor and render
A pure function always returns the same result when called again, whereas an impure function might alter data, exposing errors early on.
Example: A BookList component where, at first glance, it seems like there are no errors.
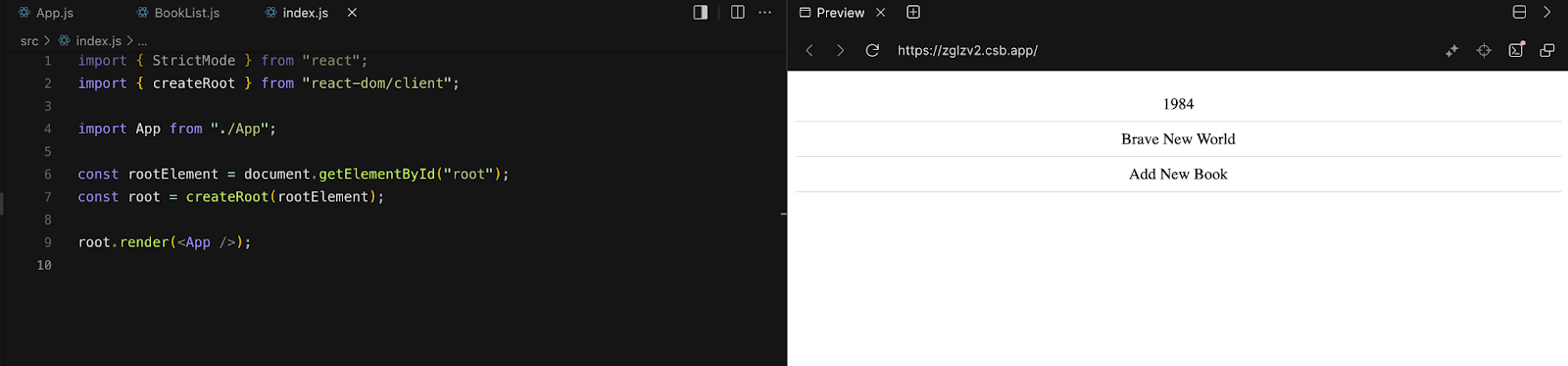
<StrictMode> is absent, and at first glance, the component seems to have no issues. But once we add <StrictMode> to our application, the error immediately becomes noticeable.
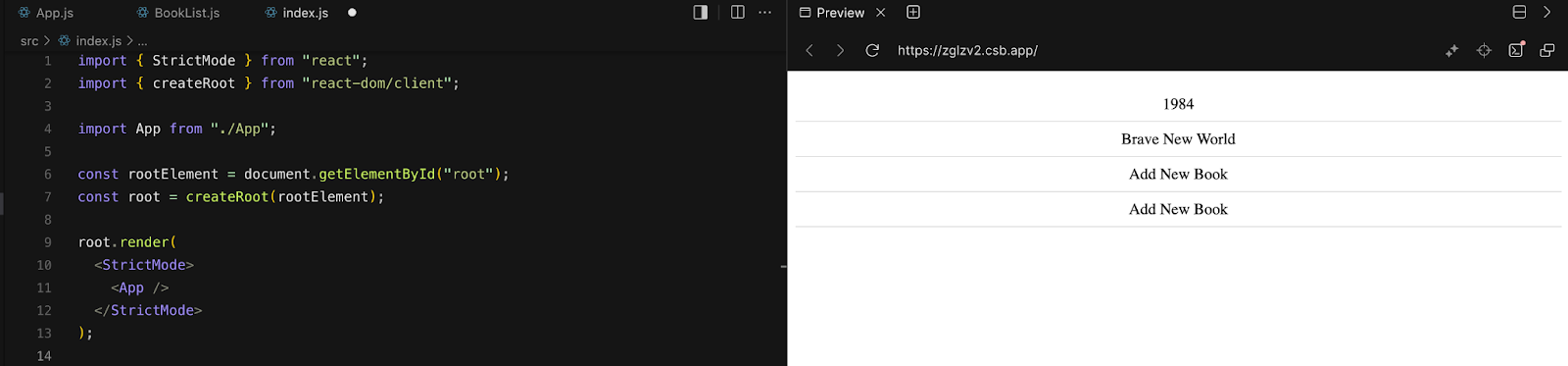
Our component is working incorrectly, and we noticed this thanks to the double rendering by <StrictMode>.
Now let's see where the error is.
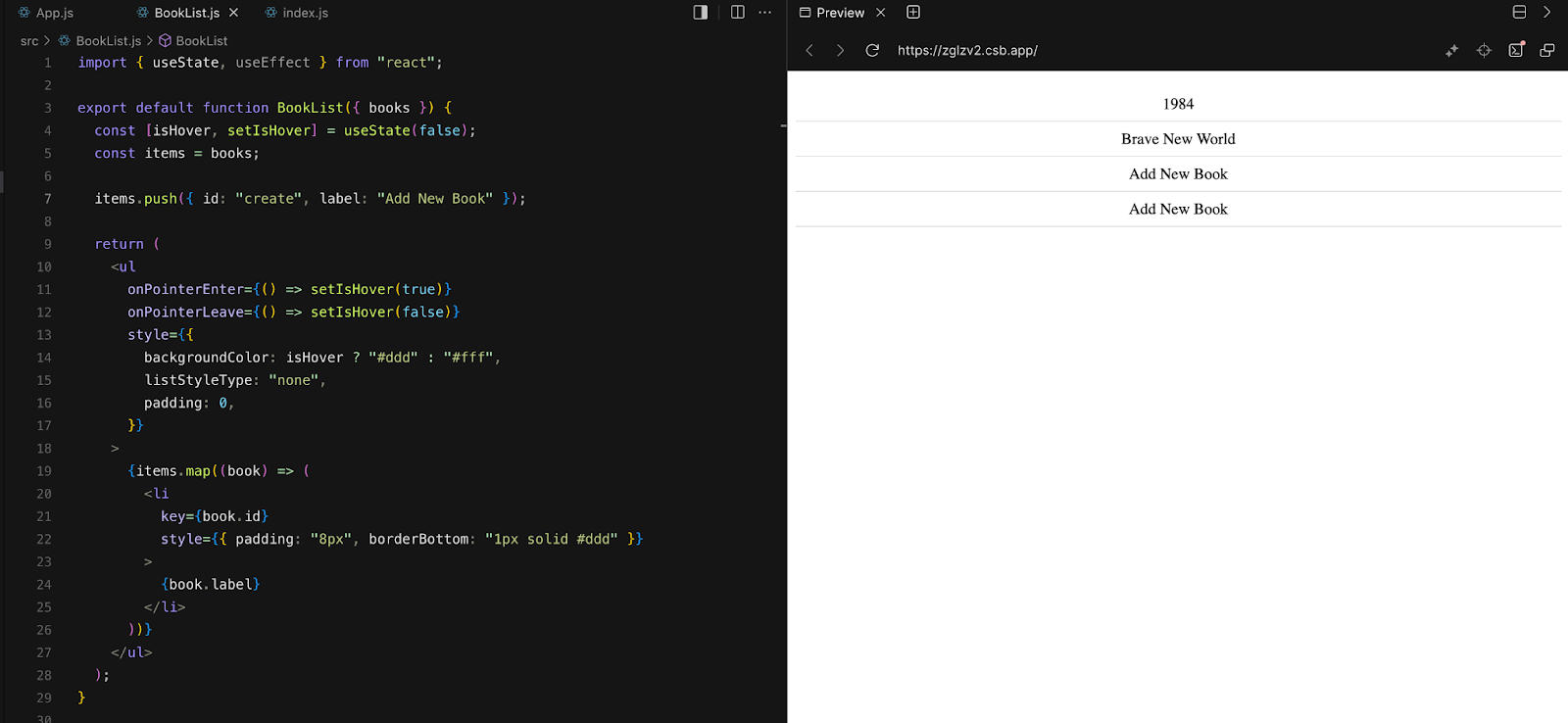
In the BookList component, we are directly modifying the books array by adding the “Add New Book” element to it. This violates React's principles of immutability, and strict mode will catch this issue due to the double rendering of components during development.
In strict mode, React calls component functions twice during development to detect side effects and improper state usage. In this case, it will result in the “Add New Book” element being added to the array twice, highlighting the problem of directly modifying the array.
To fix the error, we need to create a copy of the array before making changes.

Explanation of the Fix:
Instead of modifying the original books array directly, we create a copy of it using const items = [...books]. Then, we add the “Add New Book” element to the copied array. This prevents immutability issues and makes the component resilient to errors detected in strict mode.
Conclusion
This example demonstrates how strict mode helps to identify an error related to improper state modification within a component by forcing the component to render twice, making the issue more apparent.
like this