Understanding Pure Components in React
As we know, React application is based on function components or class components, and these React components depend on their props and state. Whenever the props or state change, React re-renders everything. However, this leads to a problem: not only is the element itself re-rendered, but all its child components are too. This occurs because React aims to keep the user interface current and may not always accurately determine which element need updating. Therefore, it re-renders all child components, even if their props haven't changed.
What should we do if we want to re-render only the type of components that actually change their states? The solution is to use Pure Components.
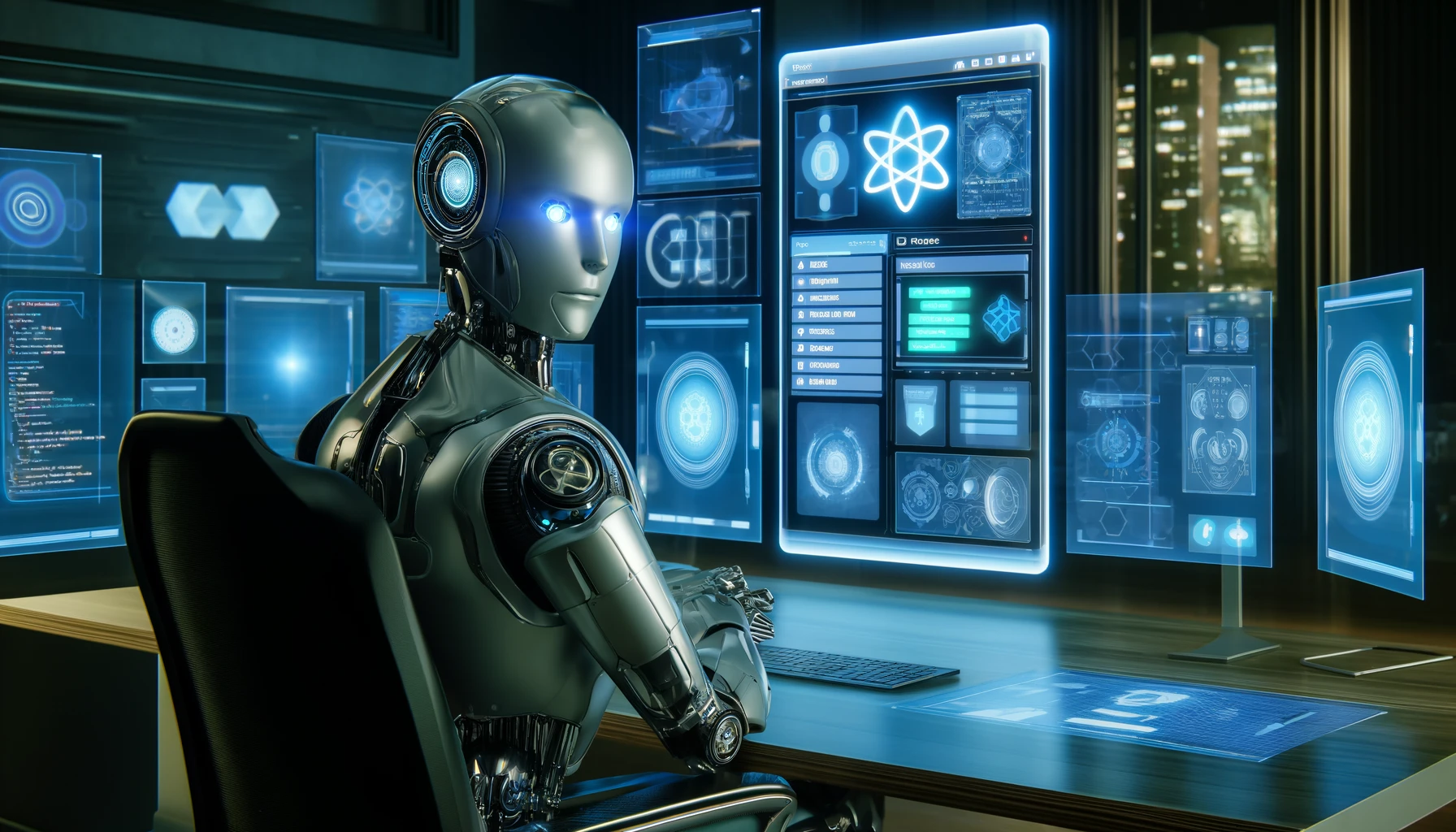
What is a pure component in React?
A React Pure Component is a component that will only be re-rendered if its props or state change. Pure components perform a shallow comparison for props and state every time they are updated. If the data remains unchanged, re-rendering will not occur. This helps conserve resources and gives application performance benefits.
According to React's principles, the rendering process should be pure. This means that regular components in React should only return their JSX without altering any objects or variables that existed before the start of rendering—modifying these would make them impure.
Let’s see what this means in a real application.
In this video, we can see that our Header component, which is purely decorative and does not change, is still re-rendered every time the parent component's state changes. To verify this, we used the useEffect hook.
Modern React development favors functional components, and React.memo is used for performance optimization of the re-rendering. Let's explore this in more detail.
React Memo
React.memo is a higher-order component (HOC) that allows for the memoization of the rendering results of a functional component. It is useful when components receive identical props and their re-rendering is unnecessary, thereby optimizing application actual performance.
How does it work?
React.memo takes a functional component as an argument and returns another component that is optimized for memoization. This wrapped component compares the incoming props with previous values, and if they are unchanged, it uses the last rendering result, thus avoiding the need to generate new JSX. Therefore, if the component's props remain the same, React.memo will skip the update process, conserving resources.
Now let's see how we can apply this to our Header component to prevent it from being re-rendered every time the parent component is updated.
As we can see, our functional component has stopped unnecessary re-renders, which caused performance improvement of our application.
Conclusion
Using React.memo in React demonstrates improved performance of your application and provides a smoother and more enjoyable user experience. Understanding how and when to apply these techniques in React is a key skill for developers looking to get the most out of dynamic web applications.
like this