String Along with JavaScript Strings
To process information, computer programs must store data as a type. A data type defines how the data is stored in memory, which operations can be applied to the data, and how to execute those operations. In computer science, string is the data type used to represent text. Strings are a fundamental part of JavaScript. They are used for storing textual data. For example, a string can store a person’s name, a book title, a movie quote, a song lyric, a poem, a paragraph, a book, a speech, a letter, a recipe, a blog post, a tweet, a comment, a text, an email, a chat message, and so on.
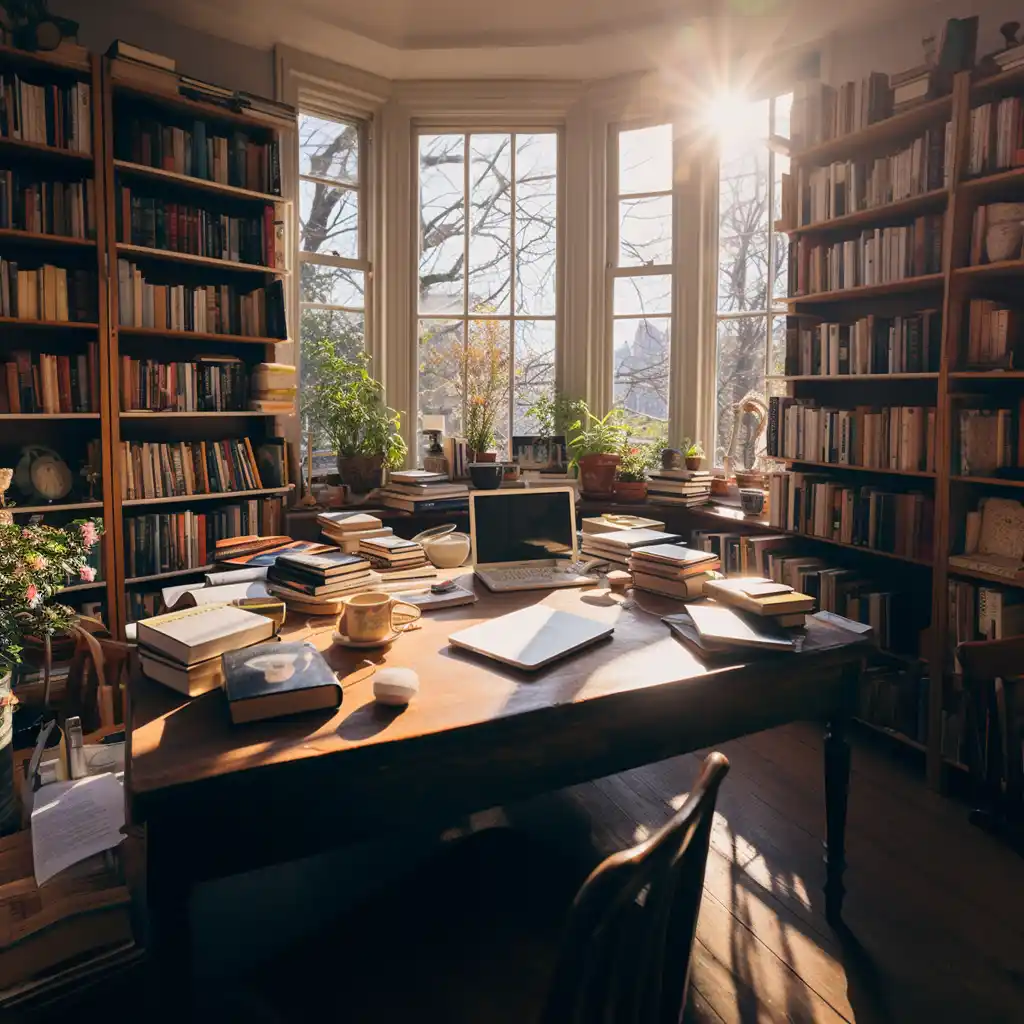
What is a JavaScript string?
In JavaScript, a string is a sequence of characters. Characters are symbols, including letters, punctuation, and various other written marks in a language. For example, a, B, c, 1, 2, 3, @, #, and $ are characters in many European languages like German or Norwegian, romance languages like French or Spanish, African Swahili, and Vietnamese.
A string can be a word:
A string can also be a sentence:
Or a string can be a paragraph from an article:
As you can see, a string can be as long as you want. It can be a single character (a), an empty character containing no characters (), your favorite emojis 🤣, 🎅, 🎆, 🎇, 🎈, 🎉, 🎄, 🍕, 🍺, 🍩, 🍪, 🍫, 🍬, 🍭, 🍮, or a whole book.
As a JavaScript developer, you must often create, manipulate, and compare strings. You may need to create strings to store textual data, convert strings to uppercase, lowercase, or title case, split a string into an array of substrings, concatenate two or more strings, search for a substring, replace a substring, reverse a string, trim a string, sort a string, compare two strings, and so on.
There are many built-in methods to work with strings. In this article, we will explore some useful string methods. However, before we do, we first need to know that there are two types of strings in JavaScript: string primitives and string objects. Let’s jump right in and explore these two types of string.
String Primitives vs String Objects
A string primitive is a string that is not an object and has no methods. A method is a function associated with an object. A string object is a wrapper around the string primitive data type. To understand these two types of strings, take a look at the following code snippet:
In the code above, stringPrimitive and anotherStringPrimitive variables are string primitives. The only difference between the two is that stringPrimitive was created using a string literal, and anotherStringPrimitive was created using the String constructor. Our last variable, stringObject, is a string object created using the String constructor. Stay calm if you need to become more familiar with the String constructor. I will explain it in more detail in this article. Meanwhile, let’s look at the difference between string primitives and objects.
String Primitives
As seen in the code above, there are two ways to create primitive strings. You can create primitive strings using string literals or the String constructor.
String literals are the most common way to create strings in JavaScript. To create a string literal, you enclose the string in single quotes ' ', double quotes " ", or backticks ` `.
The following are valid string primitives using the string literals:
The second way to create primitive strings in JavaScript is to use the String constructor. The String constructor is a built-in global object in JavaScript representing a text string.
The following are valid strings primitives using the String constructor:
Note that in the above code snippet, the String() constructor is called without a new keyword. There is a difference between calling the String() constructor with or without the new keyword. We will cover this difference in our next section, string objects.
String Objects
String objects are created using the String constructor. You can use the new keyword with the String constructor to create a string object.
The following are valid string objects using the String constructor:
Note that the String() constructor is called with the new keyword. The String constructor can be used with or without the new keyword. When the String() constructor is called without the new keyword, it returns a string primitive. However, when the String() constructor is called with the new keyword, it returns a String object.
Take a look at the flowing code snippet:
Although it is rarely used to create strings, it is essential to know that the String constructor can be used to create string primitives and string objects. You need to know that regardless of how a string primitive is made, it is still a string primitive type and has no methods. On the other hand, a string object is an instance of the String object and has many methods. With this in mind, we may be tempted to think that from the code above, stringPrimitive has no methods at all. However, this is different. As they say, the devil is in the details. Let’s take a look at the details:
Wait a mousy minute! The toUpperCase() method converts the string primitive to uppercase. Why does the toUpperCase() method work on a string primitive when string primitives have no own methods? The answer is coercion. JavaScript behind the scenes converts string primitives to string objects when a string primitive is used with a method. For this reason, you can use the String constructor methods such as toUpperCase(), toLowerCase(), charAt(), and so on as if they were string objects. JavaScript automatically coerces a string primitive to a string object if the new keyword is not used.
In summary, there are two types of strings in JavaScript: string primitives and string objects. Primitive strings can be created as string literals using single quotes ' ', double quotes " ", or backticks ` `. Primitive strings can also be created using the String constructor without the new keyword. When the String constructor is called with the new keyword, it returns a string object. JavaScript converts string primitives to string objects behind the scenes when a string primitive is used with a method. For this reason, you can use methods on string primitives as if they were string objects. Now, are you ready to have fun working with strings?
Working with Strings
As we said earlier, although primitive strings and string objects are different data types, they can be used similarly. You can use the same methods on both string primitives and string objects.
Let’s see this in action using the toUpperCase() and slice() methods:
In the above code snippet, the toUpperCase() method is used to convert the first letter of name to uppercase. The slice() method extracts the rest of the string after the first letter. The + operator concatenates the uppercase letter with the rest of the string. So, the sentence output is Hello, world, my name is John. Don't worry if you need help understanding the above code snippet, as we work with string methods in the upcoming sections. Before we do, let’s cover two critical concepts about strings: immutability and iterability. Let’s start with immutability.
String Immutability
Strings are immutable, meaning their values cannot be changed once created. String immutability is a property of strings in JavaScript that prevents them from being changed once created. It means that once you create a string, you cannot change its contents, length, or properties. However, new strings can be created based on existing ones.
There are a few ways to work around this limitation. One way is to create a new string with the modified contents. Another way is to use a string object that implements the .replace() method. We will learn about the .replace() method when we cover string methods.
Another way to work around this limitation is to use the String constructor. Recall that the String constructor can be used to create string primitives and string objects. When the String constructor is called with the new keyword, it returns a string object. String objects are mutable. They can be changed. For example, the following code snippet creates a string object called name that stores the value John:
The name variable stores a string object that can be changed. For example, the following code snippet changes the value of the name variable from John to Jane:
Here is an example of how to use the .replace() method to modify a string:
Take a look at the following code snippet:
The name variable is declared using the let keyword in the above code snippet. The let keyword is used to declare a variable that can be reassigned to a new value. The name variable is assigned the value John. The name variable is then reassigned to Jane. The output of console.log(name) is Jane. Looking at the code above, one might think that the name variable was changed from John to Jane. However, this is different. The name variable was not changed from John to Jane. The name variable was reassigned to a new value, Jane. It is because, as we said earlier, strings are immutable. They cannot be changed once they are created.
For example, the toUpperCase() method converts a string to uppercase. The toUpperCase() method is attached to the String object. It is used to act on the String object. The toUpperCase() method is called on a string and returns a new string with the uppercase letters. For example, the following code snippet calls the toUpperCase() method on the String object instance name:
The toUpperCase() method is called on the String object instance name in the above code snippet. The toUpperCase() method converts the string John to uppercase and returns a new string with the uppercase letters. The toUpperCase() method does not change the original string. It produces a new string with the uppercase letters. The original string is unchanged. It is called immutability. Strings are immutable. They cannot be changed once they are created. With this in mind, let’s take a look at string iterability.
String Iterability
First, let’s recognize that “iterability” is inaccurate. It is a made-up word to describe the ability to be iterated over. When we say that strings are iterable, we mean that you can access each character in a string individually using loops.
For example, the following code snippet iterates over the characters in the name string primitive and logs each character to the console:
The output of the above will look something like this:
String iterability is a property of strings in JavaScript introduced in ES6. In the previous code snippet, we used the for ... of loop to iterate over the characters in the string primitive. Now, with this added knowledge, let’s learn about creating strings.
Creating Strings
To create primitive strings using string literals, you enclose the string in single quotes ' ', double quotes " ", or backticks ` `.
The following are valid string literals:
Creating a string primitive using single quotes ' ', double quotes " ", or backticks ` ` is a matter of preference. The only requirement is that the opening and closing quotes must match.
The following are invalid string literals:
If you use single quotes to create a string, you must use single quotes to close the string. The same goes for double quotes and backticks. Single quotes, double quotes, and backticks are interchangeable. However, backticks are used to create template literals. We will learn about template literals in this article.
Using single quotes ' ' or double quotes " ", or backticks ` ` to create a string primitive is a matter of preference. However, some use cases exist where one method may be preferred. Let’s look at some use cases.
Single Quotes (' ')
Using single quotes is the most common way to create strings in JavaScript. It is used to create strings that are short and readable. Single quotes are also used to create strings that contain double quotes. For example, the following code snippet creates a string variable called sentence that stores the value He said, "Watch the giant fall.":
The single quotes ('') enclose the string. The double quotes ("") are used to enclose the string that contains double quotes (""). However, if you try to create a string that contains single quotes using single quotes, you will get an error:
The single quotes ('') enclose the string. The single quotes ('') are also used to enclose the string that contains single quotes ('). However, the single quotes ('') are not escaped. So, the JavaScript interpreter thinks that the string ends at the first single quote ('), and the rest is invalid JavaScript code.
Double Quotes (" ")
Double quotes ("") are also used to create short and readable strings. They are used to create strings that contain single quotes:
The double quotes ("") are required to enclose the string because the string contains words that contain single quotes ('). Because an apostrophe is used in words can't and it's, the string must be enclosed in double quotes (""). Enclosing the string in single quotes ('') would result in a syntax error:
The single quotes ('') enclose the string. Because an apostrophe is the same as a single quote ('), the JavaScript interpreter thinks the string ends at the first single quote ('), and the rest is invalid JavaScript code.
Backticks (``)
Backticks are used to create template literals. Template literals are used to create strings that contain placeholders. It is known as string interpolation. String interpolation is evaluating a string literal containing one or more placeholders. It is a feature of ES6 that allows you to insert variables into a string.
Let’s take a look at an example of string interpolation:
While the above code snippet works, there are better ways to create a string that contains placeholders. When using template literals, you should use placeholders instead of hard-coding the values. Your string should contain placeholders that will be replaced with the actual values. Your placeholders can be variables, expressions, or functions.
The following code snippet demonstrates how to use placeholders in a template literal:
In the above code snippet, the placeholders are indicated by the dollar sign and curly braces ${}. The placeholders are replaced with the value of the variables, expressions, or functions. For example, the placeholder ${noun} is replaced with the value of the variable noun, which is giant. The placeholder ${number} is replaced with the value of the variable number, which is 100. The placeholder ${getNoun()} is replaced with the value of the function getNoun(), which is giant. The first console.log(sentence) is He said, "Watch the giant fall.". The subsequent output is He said, "Watch the 100-foot giant fall." The last output is evaluated to He said, "Watch the giant fall.".
Template literals (introduced with backticks) allow for effortless string interpolation, enabling embedding expressions within strings.
Escape Sequences
In computer science, escape sequences are character combinations that have a different meaning than the characters they contain. They represent characters that are difficult or impossible to express directly. Special characters can be included in strings using escape characters, such as \n for a new line or \" for a double quote within a string.
Escape sequences are used to represent:
- A newline character
- A single quotation mark
- Certain other characters in a character constant
- Special characters, such as tabs, carriage returns, and quotation marks
Here are some examples of escape sequences in most programming languages:
- \n - newline
- \t - tab
- \r - carriage return
- \b - backspace
- \' - single quote
- \" - double quote
- \\ - backslash
In JS, the backslash (\) is used as an escape character. An escape character is a character that has a special meaning in JavaScript. For example, the single quote (') is a special character in JavaScript. It is used to enclose strings. However, if you want to use a single quote (') in a string that is enclosed in single quotes (''), you must escape it using the backslash (\).
Let’s go back to one of our earlier examples:
As we said, because an apostrophe is used in words can't and it's, the string must be enclosed in double quotes (""). However, if you still wanted to enclose the string in single quotes ('') for a particular reason, you would have to escape the single quotes ('') using the backslash (\):
In the above code snippet, the backslash (\) is used to escape the string's single quotes (''). The escape character (\) can also be used to escape double quotes ("") in a string that is enclosed in double quotes (""). For example, the following code snippet creates a string variable called sentence that stores the value He said, "Watch the giant fall.":
In the above code snippet, the backslash (\) is used to escape the double quotes ("") in the string.
Concatenating Strings
Sometimes, you may need to build a string by concatenating two or more strings. A string can be concatenated by joining together two or more strings. The + operator is used to concatenate strings. As we said earlier, a data type defines the operations that apply to the data. The + operator is used to add numbers together. However, when the + operator is used with strings, it is used to concatenate strings together. For example, the following code snippet concatenates the strings hello and world together:
As stated, we can also build strings by interpolating variables, expressions, or functions into a string using template literals.
String length Property
The String data type has a special property called length that returns the length of a string. The length of a string is the number of characters in the string. A property is a data value of an object. You may think of it as a variable attached to an object. It can be a primitive value, like a number or string, or a function, known as a method, which we will cover next. A property is accessed by using the dot (.) notation. The dot notation is known as the property accessor. It is used to provide access to the properties and methods of an object. You have seen the property accessor throughout this article. For example, each time we used the console.log() method to log a value to the console, we used the dot notation to access the log method of the console object as in the following code snippet:
The console object has a method called log that is used to log a value to the console. We can access the log method using the dot (.) notation as in console.log(). Similarly, the string length property is accessed using the dot (.) notation as in stringVariable.length.
When using the length property, remember that string indexing starts at zero. Therefore, JavaScript indexes the first character in a string at zero as in 0, 1, 2, 3, and so on. Please note that this does not mean that when we count the length of a string like John, we start counting at zero and say that the length of John is 3 as in 0, 1, 2, 3. When we count the characters in a string, we start counting at 1 as in 1, 2, 3, 4, and so on. Therefore, the length of John is 4. However, when we index the characters in a string, we index at zero, as in 0, 1, 2, 3, and so on. Indexing and counting are two different things. A string’s length is the number of characters in the string. A string’s index is the position of a character in the string. Indexing starts at zero. Counting starts at one. For example, the following code snippet returns the length of the string John and the index of the first character in the string John:
In the above code snippet, the length property gets the length of the string John. The length property is accessed using the dot (.) notation as in name.length. The length property returns the length of the string John, which is 4. The charAt() method gets the character at index 0 in the string John. The charAt() method is accessed by using the dot (.) notation as in name.charAt(0). The charAt() method returns the character at index 0 in the string John, which is J.
Note that the length property returns the number of characters in a string, not only the number of letters. As we said earlier, characters are symbols, including letters, punctuation, and various other written marks in a written language. Therefore, the length property will return the total number of characters in a string, including letters, punctuation, whitespaces, etc. The length property does count an escape sequence (one or more special characters) as one character because it has a special meaning in JavaScript.
The name variable stores the John string concatenated with the tab escape sequence (\t) and the string Doe. The tab escape sequence (\t) inserts a tab in a string. The name variable is then logged into the console. The output of console.log(name) is John Doe. The length property is then used to get the length of the string John Doe. The length property returns the length of the string John Doe, which is 8. The length property returns 8 because the tab escape sequence (\t) is counted as one character.
Note that an empty string has a length of 0. The empty string contains no characters.
However, the empty string is different from a string containing a whitespace. A string that contains a whitespace is not empty. It is a string that contains a whitespace, which is a character.
As the code snippet above shows, the space variable stores a string containing a whitespace. Suppose you created a string that stores a sentence that contains multiple whitespaces and other characters. In that case, the length property will return the total number of characters in the string, including all whitespaces and other characters.
Please note that there is also a property of the Function object called length. The length property of the Function object is a static property that returns the number of parameters in a function. The length property of the String object is a prototype method that returns the number of characters in a string. We will cover static and prototype methods in the next section. For now, know that the length property of the String object is not the same as the length property of the Function object. The length property of the String object returns the number of characters in a string. The length property of the Function object returns the number of parameters in a function.
The length property is the only property of the String data type. It is a commonly used property of the String object. Also, indexing is a widely used technique when working with strings. Therefore, it is vital to understand the difference between indexing and counting. Now, we are ready to learn about string methods.
String Methods
Methods are functions that are attached to an object. They are used to act on the object. While string methods do not change the original string, they return a new string with the changes. Some methods take arguments, and some do not. Arguments are values that are passed to a function. Arguments are used to customize the behavior of a function.
The key to a method is to know its signature. A method’s signature consists of its name, the data type it returns, and the order of the arguments. To programmatically know how many arguments a method takes, you can use the length property of the Function object as in the following code snippet:
The createUser() function takes three arguments: name, email, and password. The length property of the Function object is used to get the number of parameters in the createUser() function. The length property returns 3 because the createUser() function takes three arguments.
The String object has several methods that allow you to manipulate the string. Two types of methods are available on the String object: static methods and prototype methods. Static methods are called directly on the String object. Prototype methods are called on an instance of the String object. Let’s cover these two types of methods next.
Static Methods
When we say that static methods are called directly on the String object, we mean that they are called directly on the String object without creating an instance of the String object. That is, they are called on the String object itself. You do not need to create an instance of the String object to call a static method.
For example, the following code snippet calls the String.fromCharCode() static method on the String object:
In the above code snippet, the String.fromCharCode() static method is called directly on the String object. We did not need to create an instance of the String object to call the String.fromCharCode() static method as in the following code snippet:
The code will throw an error because the String.fromCharCode() method is not a prototype method. It is a static method. If the concept of static methods is new to you, the easier way to think about string static methods is to think of them as functions that are attached to the String object directly. You may have seen this before when working with the Math or Array objects. For example, the following code uses static methods on the Math and Array objects:
Static methods are called directly on the Math and Array objects. We did not need to create an instance of the Math or Array object to call the static methods. We just called the static methods directly on the Math and Array objects. The same goes for the String object. What you need to know when using static methods is that the name of the object is used to call the static method as in Math.random(), Math.ceil(), Math.floor(), Math.round(), Array.isArray(), Array.from(), Array.of(), and String.fromCharCode().
Prototype Methods
When we say that prototype methods are called on an instance of the String object, we mean that they are called on an instance of the String object. That is, they are called on the String object instance itself. You need to create an instance of the String object to call a prototype method.
For example, the following code snippet calls the toUpperCase() prototype method on the String object instance name:
In the above code snippet, the toUpperCase() prototype method is called on the String object instance name. We cannot reach the toUpperCase() prototype method directly on the String object as in the following code snippet:
The code will throw an error because the toUpperCase() is not static. It is a prototype method.
Whether using static or prototype methods, recall that JavaScript is case-sensitive. Therefore, the object name must be capitalized and spelled exactly as it is in the documentation. Therefore, String.fromCharCode() is different from string.fromCharCode(). The same is true that name.toUpperCase() is not the same as name.toUppercase().
Now that we’ve covered the two types of methods available on the String object, it is time to learn about some of the most commonly used string methods. Please note that this is not an exhaustive list of all the methods available on the String object. There are many more methods available on the String object. You can find a complete list of methods on the MDN web docs. With no further ado, let’s get stringing with our first string method.
at()
The at() method returns the method returns the character at the specified index in a string. The at() takes a single argument, which is the index of the character to return. The argument must be an integer value between 0 and String.length - 1 or between -1 and -String.length. If the argument is not an integer value between 0 and String.length - 1, or between -1 and -String.length, the at() method returns undefined.
Let’s learn how to use the at() method with some examples:
We see that the at() argument of the at() method can be a positive or negative integer. If the argument is a positive integer, the at() method returns the character at the specified index in the string. If the argument is a negative integer, the at() method returns the character at the specified index in the string, starting from the end of the string. If the argument is not an integer value between 0 and String.length - 1, or between -1 and -String.length, the at() method returns undefined.
charAt()
The charAt() method returns the character at the specified index in a string. The charAt() takes a single argument, which is the index of the character to return. The argument must be an integer value between 0 and String.length - 1. If the argument is not an integer value between 0 and String.length - 1, the charAt() method returns an empty string.
Let’s explore the charAt() method with some examples:
In the above code snippet, we see that the charAt() argument of the charAt() method must be a positive integer. If the argument is a positive integer, the charAt() method returns the character at the specified index in the string. If the argument is not an integer value between 0 and String.length - 1, if the specified index is out of the range, the charAt() method returns an empty string.
The difference between the charAt() and at() methods is that the charAt() takes only positive integers and returns an empty string if the index is out of range. In contrast, the at() method takes positive and negative integers and returns undefined if the index is out of range.
charCodeAt()
The charCodeAt() method returns the Unicode value of the character at the specified index in a string. The charCodeAt() takes a single argument, which is the index of the character to return. The argument must be an integer value between 0 and String.length - 1. If the argument is not an integer value between 0 and String.length - 1, the charCodeAt() method returns NaN.
Time to examine the charCodeAt() method with some examples:
We see that the charCodeAt() argument of the charCodeAt() method must be a positive integer. If the argument is a positive integer, the charCodeAt() method returns the Unicode value of the character at the specified index in the string. If the argument is not an integer value between 0 and String.length - 1, if the specified index is out of the range, the charCodeAt() method returns NaN. Also, if the argument is not passed to the charCodeAt() method, the argument is undefined and is converted to charCodeAt(0). So, the charCodeAt() is the same result as charCodeAt(0).
The charCodeAt() method can be helpful when working with Unicode characters. Unicode is a computing industry standard for the consistent encoding, representation, and handling of text expressed in most of the world’s writing systems.
concat()
The concat() method is used to join two or more strings. This method does not change the existing strings but returns a new string containing the text of the joined strings.
indexOf()
The indexOf() method returns the index of the first occurrence of a specified text in a string. If the specified text is not found, it returns -1.
lastIndexOf()
Similar to indexOf(), the lastIndexOf() method returns the index of the last occurrence of a specified text in a string. If the specified text is not found, it returns -1.
replace()
The replace() method replaces a specified value with another value in a string. It returns a new string where the specified values are replaced.
search()
The search() method searches a string for a specified value and returns the index of the match. If no match is found, it returns -1.
slice()
The slice() method extracts a part of a string and returns the extracted part in a new string. It takes two parameters: the start position (inclusive) and the end position (exclusive).
split()
The split() method splits a string into an array of substrings and returns the new array.
const colors = 'red,green,blue';
substr()
The substr() method returns the characters in a string beginning at the specified location through the specified number of characters.
substring()
The substring() method returns the characters in a string between two specified indices.
toLocaleLowerCase()
The toLocaleLowerCase() method converts a string to lowercase letters to the host’s current locale.
toLocaleUpperCase()
The toLocaleUpperCase() method converts a string to uppercase letters to the host’s current locale.
trim()
The trim() method removes whitespace from both ends of a string.
valueOf()
The valueOf() method returns the primitive value of a String object.
toString()
The toString() method returns a string representing the specified object.
fromCharCode()
The fromCharCode() method converts Unicode values to characters.
fromCodePoint()
The fromCodePoint() method returns a string created using the specified sequence of code points.
toUpperCase()
The toUpperCase() method converts a string to uppercase letters. When thetoUpperCase() method is called on a string, it returns a new string with the uppercase letters. For example, the following code snippet calls thetoUpperCase() method on theString object instancename:
Sometimes, you may need to convert the first letter of a string to uppercase. For example, you may need to convert the variable name to John. To do this, you can use the charAt() method to get the first character of the string name. Then, you can use the toUpperCase() method to convert the first letter to uppercase. Finally, you can use the slice() method to extract the rest of the string after the first letter.
Let’s see this in action:
In the code snippet above, charAt(0) is used to get the first character of the string name. The toUpperCase() method converts the first letter of name to uppercase. The slice() method extracts the rest of the string after the first letter. The + operator is used to concatenate the uppercase letter with the rest of the string. So, the output of console.log(name.charAt(0).toUpperCase() + name.slice(1)) is John.
toLowerCase()
The toLowerCase() method converts a string to lowercase letters.
includes()
The includes() method determines whether a string contains the characters of a specified string.
endsWith()
The endsWith() method determines whether a string ends with the characters of a specified string.
startsWith()
The startsWith() method determines whether a string begins with the characters of a specified string.
padEnd()
The padEnd() method pads the current string with a given string (repeated, if needed) so that the resulting string reaches a given length.
padStart()
The padStart() method pads the current string with another string (multiple times, if needed) until the resulting string reaches the given length.
match()
The match() method searches a string for a match against a regular expression, and returns the matches, as an Array object.
matchAll()
The matchAll() method returns an iterator of all results matching a string against a regular expression, including capturing groups.
normalize()
The normalize() method returns the Unicode Normalization Form of a given string.
repeat()
The repeat() method returns a new string with a specified number of copies of an existing string.
replace()
The replace() method searches a string for a specified value or a regular expression and returns a new string where the specified values are replaced.
replaceAll()
The replaceAll() method returns a new string with all matches of a pattern replaced by a replacement.
JavaScript provides a rich set of string manipulation methods that empower developers to work with text efficiently. This section delved into various string methods, exploring their functionalities with practical code examples. These are just a few of the many string methods available in JavaScript. They are all beneficial for manipulating and handling strings in different ways.
Summary
This article provides a comprehensive guide to working with strings in JavaScript. It begins by explaining a string, a sequence of characters used to represent text in programming. It then delves into the two types of strings in JavaScript: string primitives and string objects. String primitives are not objects and have no methods, while string objects are wrappers around the string primitive data type.
The article then explains how to create strings using string literals or the String constructor and the difference between these methods. It also covers the concept of string immutability, which means that once a string is created, its value cannot be changed.
The article also discusses string iterability, which means that you can access each character in a string individually using loops. It then explains how to create strings, including single quotes, double quotes, backticks, and escape sequences.
The article also covers the length property of strings, which returns the number of characters in a string. It then provides a detailed explanation of various string methods, including charAt(), charCodeAt(), concat(), indexOf(), lastIndexOf(), replace(), search(), slice(), split(), substr(), substring(), toLocaleLowerCase(), toLocaleUpperCase(), trim(), valueOf(), toString(), fromCharCode(), fromCodePoint(), toUpperCase(), toLowerCase(), includes(), endsWith(), startsWith(), repeat(), padEnd(), padStart(), match(), matchAll(), normalize(), repeat(), replace(), and replaceAll().
In summary, this article is a comprehensive guide to understanding and working with strings in JavaScript, covering everything from creation, manipulation, properties, and methods. JavaScript strings play a crucial role in web development as a fundamental data type for handling and manipulating textual information in applications and websites.
This comprehensive guide helped enhance your understanding of working with strings in the JavaScript language. If you have any questions or feedback, please contact me on Twitter. I would love to hear from you.
Stringing along, and happy coding!
Related Hyperskill Topics
like this